Accept Only Numbers on Dynamically Added Input Fields
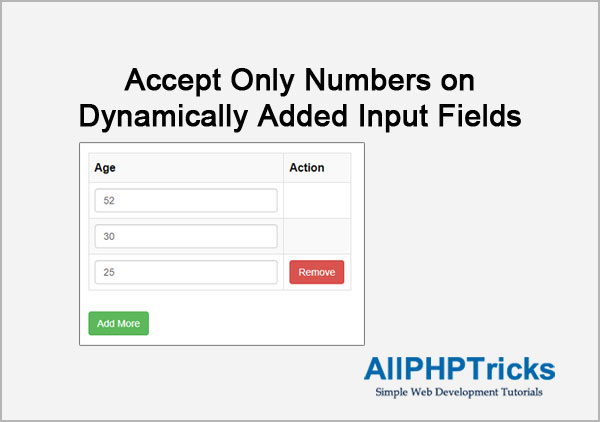
In this tutorial, I will show you how to accept only numbers on dynamically added input fields.
When you are working with forms, you may need to take input as a number, and for the client side validation, you can easily apply validation on the input fields in your form.
I have previously shared a tutorial on how to insert only numbers using jQuery. But this code will only work with those fields which are already available in the HTML form when the page is loaded.
When you need to accept only number on dynamically added input fields using jQuery, then you will need to attach the event when the new element is added dynamically to the DOM.
Steps to Accept Only Numbers on Dynamically Added Input Fields
Follow the below steps to accept only numbers on dynamically added input fields using jQuery.
- Include jQuery Library
- HTML Markup for Sample Age Field
- JavaScript for Adding Dynamic Age Input Field
- JavaScript for Accepting Only Numbers on Dynamically Added Input Fields
1. Include jQuery Library
The most important thing is to load the jQuery library in your application code.
Simply create an index.html file and copy paste the following code in before closing the body tag.
jQuery Library:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.0/jquery.min.js"></script>
2. HTML Markup for Sample Age Field
In this step, I will add an age input field in the HTML table row so that when I add new dynamically created age input field, it will be added to a same HTML table in a new row.
Just add the following HTML markup in the index.html file.
<table class="table" id="age_table">
<tbody>
<tr>
<th>Age</th>
<th style="width:96px;">Action</th>
</tr>
<tr>
<td><input type="text" name="age[]" class="numbers" id="age1" placeholder="20" maxlength="2" /></td>
<td></td>
</tr>
</tbody>
</table>
<button class="add_more">Add More</button>
3. JavaScript for Adding Dynamic Age Input Field
To explain the tutorial in a more better way, I am also showing you how to add dynamic input fields so that I can check if these dynamic fields are accepting only numbers or not.
You can also read here about how to add remove group of input fields using jQuery.
You will need to make sure this JavaScript code must come after the jQuery library.
<script>
//Add More Age Rows
$(document).ready(function() {
var max_rows = 10; //Maximum allowed input fields
var wrapper = $("#age_table"); //Input fields wrapper
var add_button = $(".add_more"); //Add button class or ID
var x = 1; //Initial input fields count
$(add_button).click(function(e){ //On click add employee button
e.preventDefault();
if(x < max_rows){ //max input fields allowed
x++; //input fields increment
$('#age_table tr:last td:last-child').html("");
$(wrapper).append('<tr><td><input type="text" name="age[]" class="numbers" id=\"age'+x+'\" placeholder="20" maxlength="2" /></td><td><button class="remove">Remove</button></td></td></tr>');
//add input fields
}
});
$(wrapper).on("click",".remove", function(e){ // On click to remove button
e.preventDefault(); $(this).parent().parent().remove(); x--;
var rowCount = $("#age_table tr").length;
if(rowCount>2){
$('#age_table tr:last td:last-child').html('<button class="remove">Remove</button></td>');
}
});
});
</script>
4. JavaScript for Accepting Only Numbers on Dynamically Added Input Fields
This is the actual JavaScript code of this tutorial which will validate that the value must be a number in dynamically added input fields.
Reader Also Read: How to Apply jQuery Datepicker on Dynamically Added Input Fields
Simply copy and paste the following JS code in an index.html in the bottom of the page after jQuery library.
// Accept Only Numbers on Dynamic Added Input Fields & Existing Input Fields
$('body').on('keypress keyup blur',".numbers", function(){
$(this).val($(this).val().replace(/[^\d].+/, ""));
if ((event.which < 48 || event.which > 57)) {
event.preventDefault();
return false;
}
});
Conclusion
I hope by now you learnt how to accept only numbers on dynamically added input fields.
By following the above mentioned steps, you can easily apply validation on dynamically added input fields to accept only numbers.
If you found this tutorial helpful, share it with your friends and developers group.
I spent several hours to create this tutorial, if you want to say thanks so like my page on Facebook, Twitter and share it.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks