Simple Laravel 10 Factory Tutorial
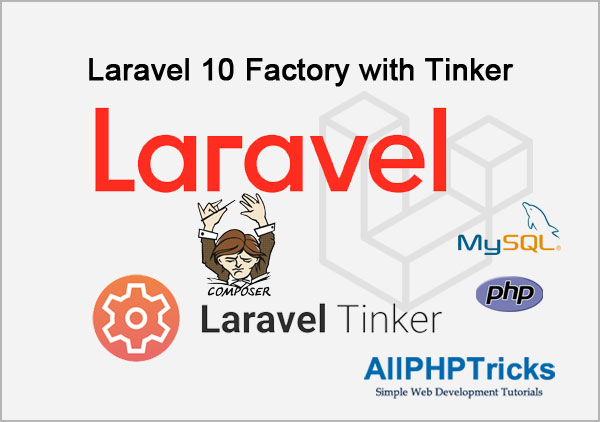
Today, in this tutorial, we will learn about Laravel Factories by creating a very simple and useful Laravel 10 Factory using PHP artisan commands with simple and easy step by step guide.
We will also use the Laravel Tinker to create records in our database table.
We are using Laravel 10 in this tutorial example. However, you can create and use Laravel Factory with Laravel’s previous versions for example with Laravel 7 Factory, Laravel 8 Factory, and Laravel 9 Factory as well.
In this tutorial, we will show you how to create a simple Laravel 10 factory with easy and simple example.
But first, you should know that what is Laravel factory and why do you need to use Laravel factory.
What is Laravel Factory?
In simple words, Laravel factory helps to insert multiple / bulk records or data into our database with easy and quickly.
When you are developing a Laravel application, you may need to insert dummy data to test the application. You can add records manually but this is a quite time consuming process. On the other hand, you can use Factory to insert thousands of records within a minute.
Laravel factories are also used in Laravel database seeders to seeding data to database with simple artisan command.
By default, Laravel comes with a sample User Factory file which is available on \database\factories\UserFactory.php
You can check this file to get the idea of how Factory file looks like and we can use this file to insert dummy users in our users table.
We will use Laravel Tinker to add records in our users table, Tinker is a Laravel package which comes with Laravel since Laravel 5.4 by default.
You can check in composer.json in “require” section, if in case it is not available in your composer then you can install it by running the below command.
composer require laravel/tinker
With Tinker we can interact with our entire Laravel application on the command line which is very powerful thing, we can interact with Laravel eloquent models, events, jobs, and many other things.
How to Use Tinker
Before start using Tinker, make sure that you have installed Laravel, configured your database credentials and migrated all your tables to database.
As we want to add records in our database so make sure that you have installed Laravel and migrated tables in database.
In order to use tinker on command line, open your terminal and run the following command to enter into Tinker environment.
php artisan tinker
When you are entered into Tinker environment then run the below command to add 10 records in your users table.
User::factory()->count(10)->create()
After that you can check your users table, it must have 10 records now.
To close tinker environment just type exit and hit enter.
So now without further delay, lets create our custom Factory for our Products table.
Steps to Create Custom Laravel 10 Factory
Follow the below simple steps to create a simple Laravel 10 Factory.
- Install the Laravel 10 App
- Configure Database Credentials
- Create a Product Model, Migration and Factory Class
- Update Product Model, Migration and Factory Class
- Migrate Tables to Database
- Add Records in Products Table via Tinker
1. Install the Laravel 10 App
Open the command prompt/ terminal and go to the directory where you want to install Laravel 10 application and then run the below command to install Laravel.
composer create-project --prefer-dist laravel/laravel:^10 laravel-factory
After installation the Laravel, go to your Laravel installation directory just by running the below command.
cd laravel-factory
2. Configure Database Credentials
Now we need to configure database credentials for Laravel 10 factory example tutorial.
Simply go to the root of our application and locate .env file and update it with our own database credentials details which are database name, database user and database password.
Database Credentials:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_db_name
DB_USERNAME=your_db_username
DB_PASSWORD=your_db_password
3. Create a Product Model, Migration and Factory Class
Following command will create a product model with migration, and product factory class.
php artisan make:model Product -mf
- –m flag is for migration
- –f flag is for a factory class
4. Update Product Model, Migration and Factory Class
Now, we need to update our product migration file, just go to the database\migrations directory and our product migration file is available there with the following name.
YYYY_MM_DD_TIMESTAMP_create_products_table.php
Just open this product migration file and copy paste the following code in it.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string('code')->unique();
$table->string('name');
$table->integer('quantity');
$table->decimal('price', 8, 2);
$table->text('description');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('products');
}
};
After that we need to go to the app\Models\Product.php file and update the product model file with the following code.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
use HasFactory;
protected $fillable = [
'code',
'name',
'quantity',
'price',
'description',
];
}
Now, it is the time to update our product factory class, just go to the database\factories\ProductFactory.php and update the product factory file.
<?php
namespace Database\Factories;
use Illuminate\Database\Eloquent\Factories\Factory;
use App\Models\Product;
/**
* @extends \Illuminate\Database\Eloquent\Factories\Factory<\App\Models\Product>
*/
class ProductFactory extends Factory
{
/**
* The name of the factory's corresponding model.
*
* @var string
*/
protected $model = Product::class;
/**
* Define the model's default state.
*
* @return array<string, mixed>
*/
public function definition(): array
{
return [
'code' => fake()->unique()->bothify('?????-#####'),
'name' => fake()->name(),
'quantity' => fake()->randomNumber(2, true),
'price' => fake()->randomFloat(2, 20, 90),
'description' => fake()->text(),
];
}
}
5. Migrate Tables to Database
After completing all steps, now we need to migrate all Laravel default tables and our product table into our database.
Run the below artisan command in terminal to migrate all tables.
php artisan migrate
And then run the below command on your terminal, otherwise you may get error in Tinker environment.
composer dump-autoload
6. Add Records in Products Table via Tinker
Now, as we have completed all the steps and migrated our tables as well. This is the time to go into the Tinker environment and create multiple records with just one command.
Run the below command on your terminal to go into Tinker environment.
php artisan tinker
And then run the below command in your tinker shell.
Product::factory()->count(100)->create()
The above command will create 100 records in products table. You can create any number of records just by changing the number in count() function in the above command.
Conclusion
We have reached to the conclusion of this Laravel 10 factory tutorial and now we hope that you have learnt how to create a simple Laravel 10 database factory with our example tutorial by following the above step by step and easy guide.
If you found this tutorial helpful, share it with your friends and developers group.
We spent several hours to create this tutorial, if you want to say thanks so like our page on Facebook, Twitter and share it.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks