How to Get the Client IP Address using PHP
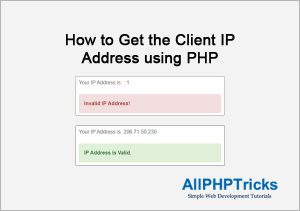
In this tutorial, I will show you how to get the client IP Address using PHP. There are various occasions when you need to get and store the client IP address in database using PHP.
Or you may also need to perform any other actions based on user’s IP address, such as blocking user for multiple invalid login attempts using IP address and others.
Readers also read: Best VPN for Web Developers to change your IP address.
Ways to Get Client IP Address in PHP
There are multiple ways to get the client IP address in PHP, I will share more than 1 methods with you.
Method 01:
PHP provides a built in super global variable $_SERVER that holds quite useful information in it. We can easily use this $_SERVER variable to get the client IP address in PHP.
The below PHP code will return the IP address of a user in PHP.
<?php
echo $_SERVER['REMOTE_ADDR'];
?>
However, the above code may give you incorrect IP address if the user is using the Proxy. But we have another solution that will resolve this issue.
Method 02:
You can use this custom PHP function which will give you the actual IP address of user in PHP even if the user is using the proxy.
This function also checks if user is using the proxy and proxy can have multiple IP addresses then it will pick the first IP address which is the most reliable IP address.
<?php
// Get the Client IP Address PHP Function
function get_ip_address() {
$ip_address = '';
if (!empty($_SERVER['HTTP_CLIENT_IP'])){
$ip_address = $_SERVER['HTTP_CLIENT_IP']; // Get the shared IP Address
}else if(!empty($_SERVER['HTTP_X_FORWARDED_FOR'])){
//Check if the proxy is used for IP/IPs
// Split if multiple IP addresses exist and get the last IP address
if (strpos($_SERVER['HTTP_X_FORWARDED_FOR'], ',') !== false) {
$multiple_ips = explode(",", $_SERVER['HTTP_X_FORWARDED_FOR']);
$ip_address = trim(current($multiple_ips));
}else{
$ip_address = $_SERVER['HTTP_X_FORWARDED_FOR'];
}
}else if(!empty($_SERVER['HTTP_X_FORWARDED'])){
$ip_address = $_SERVER['HTTP_X_FORWARDED'];
}else if(!empty($_SERVER['HTTP_FORWARDED_FOR'])){
$ip_address = $_SERVER['HTTP_FORWARDED_FOR'];
}else if(!empty($_SERVER['HTTP_FORWARDED'])){
$ip_address = $_SERVER['HTTP_FORWARDED'];
}else{
$ip_address = $_SERVER['REMOTE_ADDR'];
}
return $ip_address;
}
// Print client IP address
$ip_address = get_ip_address();
echo "Your IP Address is: ". $ip_address;
?>
Congrats, you have successfully got the IP address of your user or client.
You can easily use this function and get the client IP address using PHP. For demo purpose, I have printed the client IP address.
Reader also read: Simple User Registration & Login Script in PHP and MySQLi
Validate IP Address
It is an additional measure to check if IP address is valid or not, like if you are using the above PHP function on localhost, it will give you ::1 instead of actual IP address.
And there are some chances that you get the invalid IP from some users, so it is recommended to validate the IP address before using or saving it.
The below PHP function will check the valid IP address 4 and IP address 6. It will also validate that IP address does not fall in the range of private and reserved networks.
<?php
// Function to validate IP address and check the valid IPv4 or IPv6 address
// And it does not belong to a private and reserved range
function validate_ip($ip_address)
{
if (filter_var($ip_address, FILTER_VALIDATE_IP, FILTER_FLAG_IPV4 |
FILTER_FLAG_IPV6 | FILTER_FLAG_NO_PRIV_RANGE |
FILTER_FLAG_NO_RES_RANGE) === false) {
return false;
}
return true;
}
if(validate_ip($ip_address)){
echo "<div class='alert alert-success'>IP Address is Valid.</div>";
}else{
echo "<div class='alert alert-danger'>Invalid IP Address!</div>";
}
?>
Privacy Concern
IP address of a client is a private information and you should explicitly mention in your privacy policy that you can collecting the client IP address and mention the reason along with it.
Conclusion
I hope by now you know how to get the client IP Address using PHP. This simple PHP function will help you to get the client IP address in your web application. This is very common task in PHP applications.
If you found this tutorial helpful, share it with your friends and developers group.
I spent several hours to create this tutorial, if you want to say thanks so like my page on Facebook, Twitter and share it.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks
Hi,
Thank you for this code! Is it possible to add this code to a contact form to determine the IP of the user and write the users IP to the form results?
Hi Brian,
Yes you can use this code to any place of your application to get a client IP address.