How to Auto Select Visitors Country using IP Address in PHP
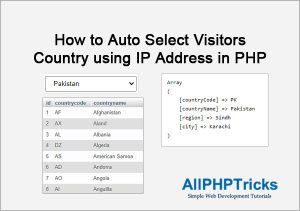
In this tutorial, I will show you how to auto select visitors country using IP address in PHP. When developing web application, you may need to automatically select your visitor’s country using PHP.
You can get the location of the visitor and select the visitor’s country using IP address in PHP.
Auto selection of country speed up the registration process if you are working on registration form.
If you are building an ecommerce website that ships a product, auto selection of country can help to display the correct shipping cost based on auto country selection.
Steps to Automatically Select Visitors Country using IP Address in PHP
There are three steps that are required to get the visitors IP address and select country using PHP.
- Create a Database Table of Country & Dump Data
- Get the Visitor IP Address in PHP
- Automatically Select the Visitor’s Country using PHP
1. Create a Database Table of Country & Dump Data
Simply copy paste the below SQL to create a table structure of country which contains country code and name.
CREATE TABLE `country` (
`id` int(10) NOT NULL AUTO_INCREMENT,
`countrycode` char(2) DEFAULT NULL,
`countryname` varchar(200) NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `countrycode` (`countrycode`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
Once the structure is created then you will need to add countries data in it. There will be more than 250 entries therefore, I have attached the SQL table in download file.
You can simply download this tutorial and import the SQL table along with countries data.
2. Get the Visitor IP Address in PHP
Previously, I have shown to you how to get the client IP address using PHP. I will use the same method to get the client IP address but with some modification to get the location details.
If you do not have any idea about getting client IP address then I would suggest you to check out my above tutorial.
Create an index.php file and paste the following function in it.
<?php
// Get a Visitor Location Details using IP Address PHP Function
function getLocationByIp(){
$client_ip = @$_SERVER['HTTP_CLIENT_IP'];
$forward_ip = @$_SERVER['HTTP_X_FORWARDED_FOR'];
$remote_ip = @$_SERVER['REMOTE_ADDR'];
$result = array('countryCode'=>'', 'countryName'=>'', 'region'=>'', 'city'=>'');
if(filter_var($client_ip, FILTER_VALIDATE_IP)){
$ip_address = $client_ip;
}elseif(filter_var($forward_ip, FILTER_VALIDATE_IP)){
$ip_address = $forward_ip;
}else{
$ip_address = $remote_ip;
}
$loc_data = @json_decode(file_get_contents("http://www.geoplugin.net/json.gp?ip=".$ip_address));
if($loc_data && $loc_data->geoplugin_countryName != null){
$result['countryCode'] = $loc_data->geoplugin_countryCode;
$result['countryName'] = $loc_data->geoplugin_countryName;
$result['region'] = $loc_data->geoplugin_region;
$result['city'] = $loc_data->geoplugin_city;
}else{
$result['countryCode'] = 'US';
}
return $result;
}
$loc = getLocationByIp();
$countryCode = $loc['countryCode'];
?>
First I am getting the IP address then passing that IP address to a geoplugin.net website which will gives us the location details, there are various websites which provides the location data based on IP.
It will return the countryCode, countryName, region and city and many other details but I will get these details for now as I will only use the countryCode.
Now I have stored countryCode value in $countryCode variable, I have a user’s country code which I will use in the step 3 below.
Readers Also Read: Best VPN for Web Developers to change the IP address to test application from other countries IP addresses.
3. Automatically Select the Visitor’s Country using PHP
First I will display all the countries from database and then check if any country code matches with the code I got from above PHP function using client IP address.
Simply copy paste the below code in index.php
<select name="country_code" class="form-select">
<?php
require_once('dbclass.php');
$db = new DB;
$db->query("SELECT `countrycode`, `countryname` FROM `country` ORDER BY `countryname` ASC");
$countries = $db->resultSet();
$db->close();
foreach($countries as $row)
{
?>
<option value="<?php echo $row['countrycode'] ?>" <?php if ($countryCode==$row['countrycode']){echo "selected";}?>>
<?php echo $row['countryname'] ?>
</option>
<?php } ?>
</select>
I am using the dbclass.php to fetch data from database, this contains a PDO to get data. I have written a separate tutorial about PDO in PHP.
Make sure that you update the $db_name = ‘database_name’ to your DB name in file dbclass.php.
This class file is added in download of this tutorial. However, if you want to learn more about PDO then check out my tutorial on PHP CRUD Operations Using PDO Prepared Statements.
Conclusion
I hope by now you know how to auto select visitors country using IP address in PHP. Getting client IP address first then pass it to an API to get the location information based on IP address.
Once you get the countryCode then you can matches the code in your database country table to auto select country based on IP address.
If you found this tutorial helpful, share it with your friends and developers group.
I spent several hours to create this tutorial, if you want to say thanks so like my page on Facebook, Twitter and share it.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks