How to Send Email in Laravel 9 using SMTP
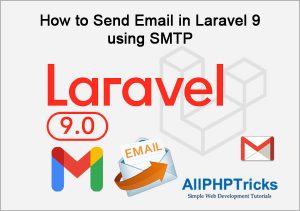
In this tutorial, I will show how to send email in Laravel 9 using SMTP with step by step guide.
Sending an email is the core functionality of any application, Laravel also comes with drivers to send email. There are many ways you can send your email through like SMTP, Amazon SES, sendmail, Postmark, and Mailgun.
In this tutorial example, I will show the step by step guide on how to send email in Laravel 9 using Gmail SMTP and your own website host SMTP.
Steps to Send Email in Laravel 9
Follow the below simple steps to send email in Laravel 9 using SMTP.
- Install Laravel 9 & Configure SMTP Credentials
- Create a Mail Class
- Create a Mail Controller
- Create an Email Directory and Blade View
- Add a Send Email Route
- Run and Testing the Application
1. Install Laravel 9 & Configure SMTP Credentials
First of all, I will install a fresh copy of Laravel 9 Framework by running the below command in command prompt or terminal.
composer create-project --prefer-dist laravel/laravel sendEmail
Although installation of Laravel 9 is not mandatory here, you can apply email sending functionality to your existing Laravel application.
Now move to your application directory by running the below command.
cd sendEmail
Once Laravel is installed successfully then I will configure SMTP and set all the credentials which will be used by Laravel to send email.
For Gmail SMTP configuration, make the changes in your .env file as below.
MAIL_MAILER=smtp
MAIL_HOST=smtp.gmail.com
MAIL_PORT=465
[email protected]
MAIL_PASSWORD=your_password
MAIL_ENCRYPTION=tls
[email protected]
MAIL_FROM_NAME="${APP_NAME}"
Note: If you are using Gmail to send your email so you will need to enable Less secure app access from your Gmail account setting from here https://myaccount.google.com/u/1/lesssecureapps.
For your own website host SMTP configuration, make the changes in your .env file as below.
MAIL_MAILER=smtp
MAIL_HOST=your_website.com
MAIL_PORT=465
MAIL_USERNAME="your_email@your_website.com"
MAIL_PASSWORD="your_password"
MAIL_ENCRYPTION=tls
MAIL_FROM_ADDRESS="your_email@your_website.com"
MAIL_FROM_NAME="${APP_NAME}"
If you do not know how to create email account on your website then follow my tutorial on how to send email in PHP using PHPMailer, I have shared detailed guide which will help you to create your new email address for Laravel configuration through Cpanel.
2. Create a Mail Class
Now, I will create a Mail class with name SendMail for sending our test email. This will call the view of test email.
Run the below command to create a Mail class.
php artisan make:mail SendMail
Now update the code on app/Mail/SendMail.php file as below.
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
class SendMail extends Mailable
{
use Queueable, SerializesModels;
public $testMailData;
/**
* Create a new message instance.
*
* @return void
*/
public function __construct($testMailData)
{
$this->testMailData = $testMailData;
}
/**
* Build the message.
*
* @return $this
*/
public function build()
{
return $this->subject('Email From AllPHPTricks.com')
->view('emails.testMail');
}
}
3. Create a Mail Controller
In this step, I will create a controller with name EmailController with an index() method that will send an email to your desired email address.
Run the below command to create a mail controller.
php artisan make:controller EmailController
Now update the app/Http/Controllers/EmailController.php code as below.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Mail;
use App\Mail\SendMail;
class EmailController extends Controller
{
public function index()
{
$testMailData = [
'title' => 'Test Email From AllPHPTricks.com',
'body' => 'This is the body of test email.'
];
Mail::to('[email protected]')->send(new SendMail($testMailData));
dd('Success! Email has been sent successfully.');
}
}
4. Create an Email Directory and Blade View
In this step, I will create an email directory in resources/views directory and then create a new email blade view file with name testMail.blade.php and paste the below code in it here resources/views/emails/testMail.blade.php.
<!DOCTYPE html>
<html>
<head>
<title>AllPHPTricks.com</title>
</head>
<body>
<h1>{{ $testMailData['title'] }}</h1>
<p>{{ $testMailData['body'] }}</p>
</body>
</html>
5. Add a Send Email Route
In this step, I will create a web route that will send testing email, add the below code in routes/web.php file.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\EmailController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/send-email', [EmailController::class, 'index']);
6. Run and Testing the Application
Now, I have completed all required steps to send an email from Laravel 9 using Gmail SMTP and your own website host SMTP. It is the time to start the development server and test the application.
Run the below command to run the development server.
php artisan serve
Now go to the browser and hit the below URL to send email from Laravel 9 using SMTP.
http://localhost:8000/send-email
You will get the email like below.
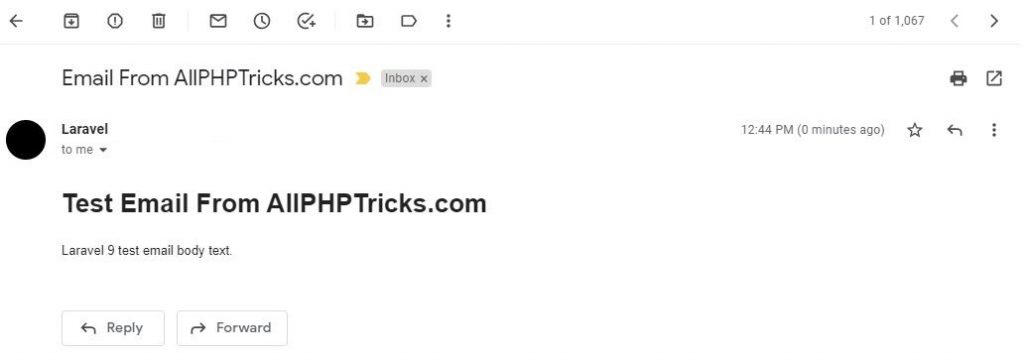
Hope this will help you to send email from Laravel 9 using SMTP.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks
Hi,
Is it possible in Laravel 9 to send an email like we send using the native PHP mail function in a simple controller function?
Thanks
Hi Amir, Laravel provides several ways to send email, native PHP mail function does not part of it. I have never tried it out, but I would recommend you to use Laravel recommended email methods to sending email. PHP native mail function is good for learning the process of sending email but it is not recommended to use in live application. You may face various issues and it does not comes with features like email methods gives you.
thank you for this great tutorial, btw you forgot to put
use App\Http\Controllers\EmailController;
inside the route web.php
Thanks for your input but it is already there, I do test the application before share the code with my readers.