Create an Accordion with HTML, CSS and jQuery
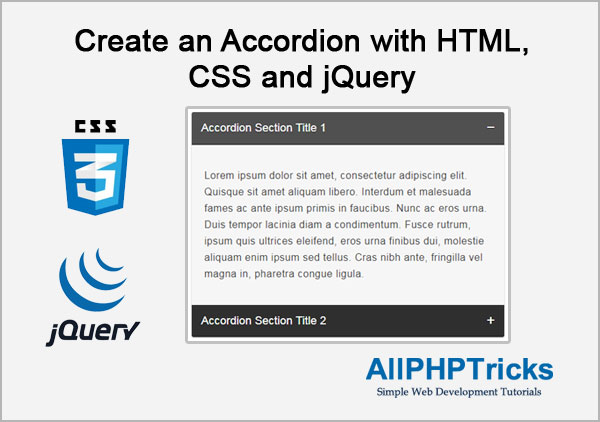
Today I will share how to create an accordion with HTML, CSS and jQuery, accordion is very common now a days, and it is very useful, you have seen it various time at FAQs section where list of questions appearing, by clicking on link its details are being displayed below the question.
You might like this Create a Popup Modal using jQuery.
Steps to Create an Accordion with HTML, CSS and jQuery
To create an accordion with HTML, CSS and jQuery, we need to follow the below steps.
- Write an HTML Markup
- Write CSS
- Write jQuery
1. Write an HTML Markup
First of all, we need to create an index.html file and paste the following HTML markup in its body section.
<div class="accordion">
<div class="section">
<a class="section-title" href="#accordion-1">Accordion Section Title 1</a>
<div id="accordion-1" class="section-content">
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Quisque sit amet
aliquam libero. Interdum et malesuada fames ac ante ipsum primis in faucibus.
Nunc ac eros urna. Duis tempor lacinia diam a condimentum. Fusce rutrum, ipsum
quis ultrices eleifend, eros urna finibus dui, molestie aliquam enim ipsum
sed tellus. Cras nibh ante, fringilla vel magna in, pharetra congue ligula.</p>
</div><!-- section-content end -->
</div><!-- section end -->
</div><!-- accordion end -->
HTML Explained
First we have created main div named accordion. All accordion sections will be inside this main div.
<div class="accordion">
</div>
After that we created a section for accordion which contain the title of accordion and content of accordion.
<div class="section">
</div>
So if you want to create multiple accordion, simply create multiple section div in main accordion div.
2. Write CSS
Second step is to write a CSS for accordion, paste the following CSS in style sheet.
body {
font-family:Arial, Helvetica, sans-serif;
}
p {
font-size: 16px;
line-height: 26px;
letter-spacing: 0.5px;
color: #484848;
}
a {
text-decoration:none;
}
/* Accordion */
.accordion, .accordion * {
box-sizing:border-box;
-webkit-box-sizing:border-box;
-moz-box-sizing:border-box;
}
.accordion {
overflow:hidden;
box-shadow:0px 1px 3px rgba(0,0,0,0.25);
border-radius:3px;
background:#f6f6f6;
}
/* Section Title */
.section-title {
background:#2f2f2f;
display:inline-block;
border-bottom:1px solid #1a1a1a;
width:100%;
padding:15px;
transition:all linear 0.15s;
color:#fff;
font-size:18px;
text-shadow:0px 1px 0px #1b1b1b;
}
.section-title.active,
.section-title:hover {
background:#505050;
}
.section:last-child .section-title {
border-bottom:none;
}
.section-title:after {
/* Unicode character for "plus" sign (+) */
content: '\02795';
font-size: 13px;
color: #FFF;
float: right;
margin-left: 5px;
}
.section-title.active:after {
/* Unicode character for "minus" sign (-) */
content: "\2796";
}
/* Section Content */
.section-content {
display:none;
padding:20px;
}
Although the CSS are self explanatory, I have also commented in CSS for your ease.
But I will explain that first I defined the style for accordion div, then accordion section title and in the last for its content. You can also see that there are + and – signs for opened and closed accordion. For that purpose, I have defined CSS for + sign in .section-title:after and for – sign in .section-title.active:after
3. Write jQuery
Don’t forget to add the jQuery Library before the below jQuery script, jQuery library file is also available in the download of this tutorial.
<script>
$(document).ready(function() {
$('.section-title').click(function(e) {
// Get current link value
var currentLink = $(this).attr('href');
if($(e.target).is('.active')) {
close_section();
}else {
close_section();
// Add active class to section title
$(this).addClass('active');
// Display the hidden content
$('.accordion ' + currentLink).slideDown(350).addClass('open');
}
e.preventDefault();
});
function close_section() {
$('.accordion .section-title').removeClass('active');
$('.accordion .section-content').removeClass('open').slideUp(350);
}
});
</script>
jQuery Explained
We created a function close_section() to remove .active class from section title and remove .open class from section content.
Whenever user clicked on any section title, function close_section() will be triggered and if user clicked on new section title then we will also add .active class and .open class to section title and section content respectively.
Conclusion
Creating an accordion with HTML, CSS and jQuery is very easy, you can add multiple section in your accordion, all you need to do is change href=“#accordion-1″ value of section title and div id=“accordion-1″ value, both value (section content div and section title) should be same.
If you found this tutorial helpful, share it with your friends and developers group.
I spent several hours to create this tutorial, if you want to say thanks so like my page on Facebook and share it.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks