Upload File Using PHP and Save in Directory
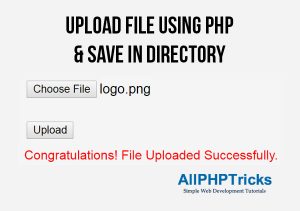
Uploading file is one of the most common feature which we normally use in our daily life, we do upload pictures on Facebook, Twitter and other websites. So today I will share a tutorial about how to upload file using PHP and save/store that file in your web server directory.
HTML Form
<form name="form" method="post" action="upload.php" enctype="multipart/form-data" >
<input type="file" name="my_file" /><br /><br />
<input type="submit" name="submit" value="Upload"/>
</form>
We will need a form with one input field with file type. The action tag of form should point to the URL of PHP script file which actually perform the uploading task. It is also important to add enctype=”multipart/form-data” property in your form to upload file otherwise your file will not be uploaded.
PHP Script
if (($_FILES['my_file']['name']!="")){
// Where the file is going to be stored
$target_dir = "upload/";
$file = $_FILES['my_file']['name'];
$path = pathinfo($file);
$filename = $path['filename'];
$ext = $path['extension'];
$temp_name = $_FILES['my_file']['tmp_name'];
$path_filename_ext = $target_dir.$filename.".".$ext;
// Check if file already exists
if (file_exists($path_filename_ext)) {
echo "Sorry, file already exists.";
}else{
move_uploaded_file($temp_name,$path_filename_ext);
echo "Congratulations! File Uploaded Successfully.";
}
}
As you can see above I tried to use the variable name as its function so that you can easily understand what I am doing, I just check that if file already exist it will print the error otherwise file will be uploaded.
This tutorial is only for the basic learning purpose, if you want to implement it on your website so it is better to use more validation before uploading file.
//you can easily get the following information about file:
$_FILES['file_name']['name']
$_FILES['file_name']['tmp_name']
$_FILES['file_name']['size']
$_FILES['file_name']['type']
Validation such as file type (extension) or file size can also be check on the client side I also wrote tutorial Check File Size & Extension Before Uploading Using jQuery.
If you found this tutorial helpful so share it with your friends, developer groups and leave your comment.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks
Sir your help is required ,please help me . I have a file.php page on which i put one and
I want to select a file may be .pdf or .doxc from my local folder and on button click it will be display on the same page below the button click without uploading it in to db. Please help me….
Dear Santosh, file will be uploaded on your server directory and you can save file record in your DB.
It’s very helpful for me. Thank you sir.
You are welcome Pawan.
Hi , i’m trying to make automatic upload instead user selects a file, , please help me thanks.
Dear Khalid,
Currently, I did not publish any tutorial which can help you out, but I hope I will try my best to make one.
I am trying to use your code but when I click the submit button it just opens a tab with the PHP script. How do I fix this? just a beginner sorry.
Dear Joseph,
IF you are beginner, then first you will need to download xampp on your PC then you will need to download the code of my website and paste into the htdocs folder and run Apache on XAMPP. You can also check how to Install and run PHP script here https://www.allphptricks.com/how-to-run-php-files-on-localhost/
is there a way to make something like this without php? because i know html and javascript it would be easier.
Well uploading file on server can be done via server side language only, you can use PHP or any other server side language.
Hi, I want to know how to upload multiple videos in a specific category and store in the specific folder category. So each file will be store in specific folder, so it will be easy to manage folders and files.
Hi Sami, you will need to provide a drop-down list at the time of video uploading, based on selected category, you can change the location where you want to upload file, you can check on server side the category id and based on id change the folder name so that file will be uploaded in that specific folder.
Hello Sir Javed your code worked perfectly ! but after saving the file in our folder I need a way of reading it and sending the data back to the client side . I am saving a .iif file which is a quickbook extension . How can I achieve my goal of reading an iif file in php and sending data as an array
Dear Haris,
You will need to create a program that will allow to read such file types.
I have:
Warning: Undefined array key “my_file” in C:\xampp\htdocs\Thales\upload.php on line 2
Warning: Trying to access array offset on value of type null in C:\xampp\htdocs\Thales\upload.php on line 2
What is wrong ?
Make sure that you have added this enctype=”multipart/form-data” in the form.
use error_reporting(0);
I am sure that your problem will be solve
Hi,
Ubuntu 18.04.5
LAMP – PHP 7.2
Used your pack changing the upload directory path. Given directory permissions with ‘chmod’. On browsing, the index page showing properly (http://localhost/filesupload) file selection is also working. But when I click the upload button it’s redirecting to a blank page, nothing happening (in browser path: http://localhost/filesupload/upload.php). Is there I am missing anything in the configuration?
If you are using the similar code so there should be no problem. However, if you are still facing error, you will need to debug the code via printing the value of submitted by form.
hi! thanks for this tutorial, but can you also show me an example of how to view what I downloaded?
Dear Javed
I think the reason why the uploaded file wouldn’t show in the uploaded folder because you haven’t put a php.ini which allows files to be uploaded file_upload=on
Thanks levi
Yes it is server configuration which is normally on, if it is not then you will need to ask your hosting provider to enable it.
cool
Indeed, useful tutorial.
Couple of things to focus if you are getting an error: HTML Form always needs to be “Post” method and You should create “Upload” folder manually, else uploading will not work well.
How can i upload the image into the database and retrieve it back again using php?
File is not visible inside upload folder in server. What is the problem.
Hi nice work but i need that when i am uploading, i can select a folder in which to upload. i have different file to upload to different folders
Well common practice is that we never tell the user that in which location we are upload files for security reasons but if you want to build application for your custom limited users, you can add additional field with options of location and based on these location selection, upload the file in the selected location.
Hello guys , why it gives me the message that file was uploaded but I don’t find it on my web site folder where I wanted it to store. When I do on windows it works very so well why it can’t on my website online.
Make sure that file uploading function is enabled on your server.
Notice: Undefined index: my_file in C:\xampp\htdocs\test\upload.php on line 13
Notice: Trying to access array offset on value of type null in C:\xampp\htdocs\test\upload.php on line 13
getting this error
Warning: move_uploaded_file(upload/BSError.PNG): failed to open stream: No such file or directory in C:\xampp\htdocs\test\upload.php on line 28
Warning: move_uploaded_file(): Unable to move ‘C:\xampp\tmp\php6FCE.tmp’ to ‘upload/BSError.PNG’ in C:\xampp\htdocs\test\upload.php on line 28
Nice one Javed.
Thanks for the appreciation.
Hi, i try upload photo with 3mb size but can’t copy to the directory. Any suggest ?
Increase size of uploading via PHP.ini file in xampp or wampp. You can watch tutorial on youtube by searching how to increase PHP max file size.
Thanks
Nice code
Thanks Ahmed
Well done my friend.. i achieved to implement to my existent project. P.S Guys, first just do quick a new project foowing his advices, and then adapt to your… there is no where a full website to copy/paste. Get info, if it works, folow the guy, and put your brain to work. 3 EXAMPLES TOOK FROM HIM UNTIL NOW, AND IT WORKS GREAT IN REAL PROJECTS!
Glad to see that you found it helpful.
Bhai Pls the CMS processing in PHP JQUERY MYSQLI etc
Notice: Undefined index: my_file in D:\xampp\htdocs\upload\upload.php on line 2
Sir I’m getting this error when I run the code. What to do?
Please reply soon.
You need to make sure if form is submitted then check file, simply download tutorial from the download button and execute it in your localhost or live host.
I am pretty sure, you are not checking that form is submitted or not.
Can this be used also to insert the files to the database as well.?
Hi Isaiah, we can not insert file is database, files are always uploaded in directory in your web host and that file location/address is stored in database.
Nice tutorial
Thanks Thavamani
Do you have coding php for upload and display image file into mysql database? I need guidance for database connection for this method.
Images are uploaded in Host like you upload other files and logo etc. But the location of uploaded image is stored in database, so it could be something like that for example: images/upload/imagename.jpg, it will be store in database and file will be uploaded in your host not in database, hope this will help you.
sir code for delete image when edit data
Use PHP unlink function to delete image or any file from server.
Could the reason why it not appearing in the folder once uploaded could be the .htacess file won’t allow it
what should be inside of upload.php?
PHP script mentioned above should be in uploaded.php
Thanks for sharing this wonderful tutorials. I was wondering if you could elaborate or expound some more on the variation of the code if you wanted to upload multiple pictures to the same record in the database? Thanks in advance, Chris
the upload folder still empty, maybe the code is not working
Make sure that you are using enctype=”multipart/form-data” also make sure that you are testing this script either on server or if you are using it on local machine then files must be your localhost, such as Apache or any other server.
There’ѕ definitely a lot to know about this issue.
I really like alⅼ the points you have made.
how i see the upload file name in url path?
Not Working so Check Ur Coding and Publishing after the testing
Hello Friend..
To some way to make the Upload that is done be saved at home different login account .. And that each person can see what put in the upload.
hi Javed, can u help me here, how do I display those uploaded files to the body of html
This code is not working in WordPress!
Hi Javed,
nice article.
Would you mind checking your code. The code is not working as expected.
I don’t see any declaration of ‘my_file’
Hi Absar,
I have tested code, it is working fine. And you can see that my_file is the file name, as you can see in the HTML section.
name=”my_file”
If you are still facing problem, kindly explain more about your issue.