Simple User Registration & Login Script in PHP and MySQLi
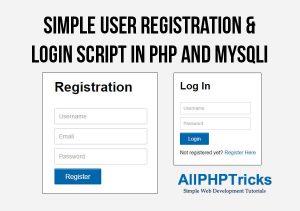
Today I will share Simple User Registration & Login Script in PHP and MySQLi, I will explain the basic functionality of registration and access secured area. In this tutorial user can register, login to member secured area and logout.
I have tested this tutorial code from PHP 5.6 to PHP 8.2 versions, it is working smoothly on all PHP versions. However, I would recommend to use the latest PHP version.
Readers Also Read: Laravel 10 User Roles and Permissions
Steps to Create Simple User Registration & Login Script in PHP and MySQLi
- Create a Database
- Create a Database Table with Following Five Fields:
id, username, email, password, trn_date - Create a Registration Form
- Create a Login Form
- Connect to the Database by using PHP
- Authenticate Logged in User
- Create Index Page
- Create Dashboard Page
- Create a Log Out
- Create a CSS File
1. Create a Database
To create a database, login to phpmyadmin and click on database tab, enter your database name and click on create database button or simply execute the below query. In my case i created database with the name register.
CREATE DATABASE register;
2. Creating a Database Table
To create table, execute the below query in SQL.
CREATE TABLE IF NOT EXISTS `users` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(50) NOT NULL,
`email` varchar(50) NOT NULL,
`password` varchar(50) NOT NULL,
`trn_date` datetime NOT NULL,
PRIMARY KEY (`id`)
);
3. Creating a Registration Form
Simple create a page with name registration.php and paste the following code in it.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Registration</title>
<link rel="stylesheet" href="css/style.css" />
</head>
<body>
<?php
require('db.php');
// If form submitted, insert values into the database.
if (isset($_REQUEST['username'])){
// removes backslashes
$username = stripslashes($_REQUEST['username']);
//escapes special characters in a string
$username = mysqli_real_escape_string($con,$username);
$email = stripslashes($_REQUEST['email']);
$email = mysqli_real_escape_string($con,$email);
$password = stripslashes($_REQUEST['password']);
$password = mysqli_real_escape_string($con,$password);
$trn_date = date("Y-m-d H:i:s");
$query = "INSERT into `users` (username, password, email, trn_date)
VALUES ('$username', '".md5($password)."', '$email', '$trn_date')";
$result = mysqli_query($con,$query);
if($result){
echo "<div class='form'>
<h3>You are registered successfully.</h3>
<br/>Click here to <a href='login.php'>Login</a></div>";
}
}else{
?>
<div class="form">
<h1>Registration</h1>
<form name="registration" action="" method="post">
<input type="text" name="username" placeholder="Username" required />
<input type="email" name="email" placeholder="Email" required />
<input type="password" name="password" placeholder="Password" required />
<input type="submit" name="submit" value="Register" />
</form>
</div>
<?php } ?>
</body>
</html>
4. Creating a Login Form
Create a page with name login.php and paste the following code in it.
<?php
session_start();
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Login</title>
<link rel="stylesheet" href="css/style.css" />
</head>
<body>
<?php
require('db.php');
// If form submitted, insert values into the database.
if (isset($_POST['username'])){
// removes backslashes
$username = stripslashes($_REQUEST['username']);
//escapes special characters in a string
$username = mysqli_real_escape_string($con,$username);
$password = stripslashes($_REQUEST['password']);
$password = mysqli_real_escape_string($con,$password);
//Checking is user existing in the database or not
$query = "SELECT * FROM `users` WHERE username='$username'
and password='".md5($password)."'";
$result = mysqli_query($con,$query) or die(mysqli_error($con));
$rows = mysqli_num_rows($result);
if($rows==1){
$_SESSION['username'] = $username;
// Redirect user to index.php
header("Location: index.php");
exit();
}else{
echo "<div class='form'>
<h3>Username/password is incorrect.</h3>
<br/>Click here to <a href='login.php'>Login</a></div>";
}
}else{
?>
<div class="form">
<h1>Log In</h1>
<form action="" method="post" name="login">
<input type="text" name="username" placeholder="Username" required />
<input type="password" name="password" placeholder="Password" required />
<input name="submit" type="submit" value="Login" />
</form>
<p>Not registered yet? <a href='registration.php'>Register Here</a></p>
</div>
<?php } ?>
</body>
</html>
5. Connect to the Database
Create a page with name db.php and paste the below code in it.
<?php
// Enter your Host, username, password, database below.
// I left password empty because i do not set password on localhost.
$con = mysqli_connect("localhost","root","","register");
// Check connection
if (mysqli_connect_errno())
{
echo "Failed to connect to MySQL: " . mysqli_connect_error();
}
?>
6. Authenticate Logged in User
Create a page with name auth.php and paste the below code in it.
<?php
session_start();
if(!isset($_SESSION["username"])){
header("Location: login.php");
exit(); }
?>
7. Creating Index Page
Create a page with name index.php and paste the below code in it.
<?php
//include auth.php file on all secure pages
include("auth.php");
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Welcome Home</title>
<link rel="stylesheet" href="css/style.css" />
</head>
<body>
<div class="form">
<p>Welcome <?php echo $_SESSION['username']; ?>!</p>
<p>This is secure area.</p>
<p><a href="dashboard.php">Dashboard</a></p>
<a href="logout.php">Logout</a>
</div>
</body>
</html>
8. Creating Dashboard Page
Create a page with name dashboard.php and paste the below code in it.
<?php
include("auth.php");
require('db.php');
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Dashboard - Secured Page</title>
<link rel="stylesheet" href="css/style.css" />
</head>
<body>
<div class="form">
<p>Dashboard</p>
<p>This is another secured page.</p>
<p><a href="index.php">Home</a></p>
<a href="logout.php">Logout</a>
</div>
</body>
</html>
9. Creating a Log Out
Create a page with name logout.php and paste the following code in it.
<?php
session_start();
// Destroying All Sessions
if(session_destroy())
{
// Redirecting To Home Page
header("Location: login.php");
exit();
}
?>
10. Creating a CSS File
Create a page with name style.css and paste the below code in it.
body {
font-family:Arial, Sans-Serif;
}
.clearfix:before, .clearfix:after{
content: "";
display: table;
}
.clearfix:after{
clear: both;
}
a{
color:#0067ab;
text-decoration:none;
}
a:hover{
text-decoration:underline;
}
.form{
width: 300px;
margin: 0 auto;
}
input[type='text'], input[type='email'],
input[type='password'] {
width: 200px;
border-radius: 2px;
border: 1px solid #CCC;
padding: 10px;
color: #333;
font-size: 14px;
margin-top: 10px;
}
input[type='submit']{
padding: 10px 25px 8px;
color: #fff;
background-color: #0067ab;
text-shadow: rgba(0,0,0,0.24) 0 1px 0;
font-size: 16px;
box-shadow: rgba(255,255,255,0.24) 0 2px 0 0 inset,#fff 0 1px 0 0;
border: 1px solid #0164a5;
border-radius: 2px;
margin-top: 10px;
cursor:pointer;
}
input[type='submit']:hover {
background-color: #024978;
}
Note: In this tutorial CSS file is placed in css/style.css folder. So make sure that you also placed CSS file in the same css folder.
Click here to Insert, View, Edit and Delete Record from Database Using PHP and MySQLi
Click here to Forgot Password Recovery (Reset) using PHP and MySQL
This is a basic PHP user registration and login tutorial, this is only for the basic concept of how user registration and login system works.
You will need to implement more security measures and validations to use this on production / live environment.
If you can understand the above tutorial, then you should learn the Laravel, I have shared a tutorial on Laravel 10 custom user registration and login.
If you found this tutorial helpful so share it with your friends, developer groups and leave your comment.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks
I tried your code on a local server and it works but when I replicate the same code to test on a staging server by only changing the db.php parameters it seems the login page can’t redirect to the index.php page. What would you think is the cause as it gives username/password is incorrect even though the username and password is correct. Any suggestion?
Alice, this is because of session. You might need to use ob_flush() in the beginning of the code.
Great script! I’m glad I found this, as I’m new to PHP and was looking for a simple user registration and login system. This script is easy to follow and does exactly what I need. Thanks for sharing!
Hey there!
after my last post today i’ve searched for a solution and i’ve found a solution for me!
Looking on “https://stackoverflow.com/questions/12525251/header-location-not-working-in-my-php-code” i’ve found any ways, but only the way with “ob_start();” in TOP of pages “auth.php” and “login.php” are working!
auth.php
========
login.php
=========
…
I do not know why, further looking using google for understanding.
Bye!
Hey Javed,
thank you for this tutorial „Simple User Registration & Login Script in PHP and MySQLi“!
Now i’m understanding the way to use MySQLi in PHP.
But i think there is a mistake in login.php.
For testing i opened registration.php, have done a registration and this works fine.
I’ve checked the database in phpMyAdmin, all data seems tob e correct.
But if i do a correct login there comes nothing, no „Welcome…“ from index.php an no „…incorrect“ from login.php an no other errormessage. Only a blank page from login.php!
It seems that the line „header(“Location: index.php”);“ in login.php doesn’t work.
Testing with incorrect user/password works fine, there comes the Message „…is incorrect“.
For testing i use EDGE an CHROME with the same result.
I’ve checked all filenames and looked many times for wrong scriptcode, all seems to be o.k.
Pls can you check your scripts to find the mistake?
If needed i can send you the scripts i used for testing.
With URL i’ve the problem, that i’ve no security with .htaccess in the moment, so you can read the data fort the database.
Sending the URL will be a risk!
So i hope you can help me..
Have a nice day!
Dear Heinz,
Just move the header location to the top of the page or use ob_flush() function to eliminate the header already sent error.
Hello Javed,
any minutes ago i leaved a comment here.
On looking for my comment i’ve seen, that an adminstrator will chek fpr publishing.
Here a solution for me, found at “https://stackoverflow.com/questions/12525251/header-location-not-working-in-my-php-code”
In auth.php and login.php i’ve added “ob_start();” in top of the page. I don’t know why, but it works!
Bye!
Hello! Great tutorial 🙂
I just have an issue, after the user registration and verifying that the user is registered on database I login on login.php that will take me to the index.php and It does but with a blank page with no error. Any hints?
Thanks
🙂
Dear Rogaros, thanks for your appreciation. Make sure that you put the session_start() at the top of your php file, right after php open tag.
Tried to use it and got this message.
Failed to connect to MySQL: Access denied for user ‘root’@’localhost’ (using password: NO)
How can I resolve it please
Dear Charles,
Update your database credentials here db.php. This is the error that your provided database credentials are incorrect.
Thanks so much! Incredibly helpful example for beginners to learn from!
Thanks Harry
Hi! after i click in login button, the page login.php is blank. what is this?
Make sure that your database is connected.
please also add remember me checkbox in login page..
if($result){
echo ”
You are registered successfully.
Click here to Login“;
}
}else{
?>
getting issue in this part when i try to enter details and make registration it shows nothing a blank page opens up, facing problem here, reply me asap
Make sure that your database connection is working fine, also check if your registered user is inserted in your database or not.
Warning: session_start(): Cannot send session cookie – headers already sent by (output started at /storage/ssd2/379/3566379/public_html/display/auth.php:7) in /storage/ssd2/379/3566379/public_html/display/auth.php on line 9
Warning: session_start(): Cannot send session cache limiter – headers already sent (output started at /storage/ssd2/379/3566379/public_html/display/auth.php:7) in /storage/ssd2/379/3566379/public_html/display/auth.php on line 9
Warning: Cannot modify header information – headers already sent by (output started at /storage/ssd2/379/3566379/public_html/display/auth.php:7) in /storage/ssd2/379/3566379/public_html/display/auth.php on line 11
Merely wanna say that this is handy , Thanks for taking your time to write this.
Great tutorial! Helpful for me. Thank You
You welcome, share it with your friends 🙂
thank you sir can i have the tutorial to delete user?thanks
Actually, i am a teacher to teach web technology this is really amazing and very easy code to understand and mainly many student easily does this project and understood well
Thanks Gopi.
Good evening
This is nice, i have a issue where i submit and the page just goes blank and nothing gets insert into the SQL
Some up please!!
Make sure your database connection is established.
Hi, how if your system is growing and have 10k-50k users, will you experience performance issue with your retrieving query/ DB design for the login part as your pk is user id only?
Hi!,I really like your writing so a lot! share we communicate more approximately your article on AOL? I need a specialist on this area to unravel my problem. Maybe that is you! Taking a look forward to peer you.
Hi! I have placed the style.cc in the /css/ folder but the css file doesn’t seem to work.
File extension must be .css so kindly make correction in style.css
While using Safari, when clicking in the first field in registration.php, it logs in instead of giving the opportunity to fill the following field. Why?
Well there is nothing additional script which may arise when using different browser, i wounder i never saw something like this before in my code. I will be better if you can share any live URL if you have so that we can have a look on it.
Hi, maybe a stupid question, but is simply setting $_SESSION[‘username’] secure? What if a malicious person would brute-force try setting $_SESSION with random usernames, and it finds a real username, it could just login bypassing password check?
HI Laurens, there are many security measures that you should take, purpose of this tutorial is to keep it simple so that everyone can understand how it works, this is not for production use, you will need to do more security checks to implement any user registration system.
Great tutorial!
my data is not getting stored in registration form plzz help
Check your database connection file, make sure that you have updated all credentials in your db.php file.
Hello Javed This is really helpful!
However I have something that I think you can help me out with, How can I can I get the data that imported to my server in my email as a list of all the registration.
Thank you!
Well if you have data in sql or excel file then you can easily import or export it from one server to another server.
Can u share a coding on inventory system of ict equipment in hospital.
Dear Nural, i am afraid that i do not have any such system right now, but may be if i get some spare time i will share some helpful tutorial related to it.
The header redirection tag to the index page does not work. I have no clue why.
To check redirection code, run it on blank page.
why my coding appear like this :
Error in inserting data due to Column count doesn’t match value count at row 1
Check column in your database table and in your query. There is either miss match of column count or you may be skipping comma (,) or (`) in your sql query, check your query clearly.
Hi, great work everything works, but I would like to add an image. In my db I have the path to it (i’m working on localhost). But when i try to display it on my webpage, the broken image appears.
If you could help me a bit
Hi Andrej, thanks for liking my tutorial.
Kindly check these things
1. Make sure your image file exist in your image file location
2. Check your image file extension is correct.
3. Also check url of image in your source code, copy and paste the image url in new tab to view is image viewing in new tab or not. If image is not working in new tab then you must check is file really exist in that directory.
Hope this help you.
I wanna tell that this is very beneficial, thanks for taking your time to write this.
while testing, my error message “incorrect username/ password” doesn’t show at the top of my form, but instead, it shows on a new page. please help
Well as you can see i am checking it on same page, so it is not possible to open this message on new window, however there may be some virus at your system which is opening new tab. I suggest you to register with simple user and password, also make sure that your registration data is in database.
Thank you so much sir for the demo code…..its perfect.
I am having a challenge with phpmailer, i edited the code that before registration details are stored unto my db, i need to verify the user email…plz help
Thank you so much for this code which is very easy to follow up and understand.
Thanks Shruti 🙂 share it with your friends.
Hi my dear friend,
i’ve been having an issue, when i try to login i put my user and pass but it does not do anything, i already checked my connection to DB and it’s all right.
Thanks Javed Ur Rehman, your code really helped me a lot.
You are really a mentor.
Thanks Prince 🙂
The login box is saying username/password incorrect, and all details are correct
Excellent your script my friend
nice tutorial regarding login system it is very helpful to my project
the form does not let me register anyone,it takes me to blank page
Kindly make sure that you are using PHP ver 5.5 not 7, i did not check this code on PHP 7.
Why do not I keep my user information in a database?
Hello,
I’ve read in many places not to use md5, being to easy to reverse process, therefore giving access to the database content to people with bad intentions. What about salt?
Also, some suggest using mysqli_real_escape, while others suggest PDO, and MANY others suggest using both. No protection is 100% injection safe. What do you think?
And as many have mentionned, it would be nice to check if the user already exists, followed by an offer to re-send the validation email.
Thanks for the follow up
I do agree with you but my tutorial is not focus for real implementation, this is only for basic concept, yes there are many other validations are needed but i did cover some validations on other tutorial. You can check out here.
https://www.allphptricks.com/check-file-size-extension-uploading-using-jquery/
My tutorials are only for basic concept purpose not for production environments.
Thank you so much ….. this is the best code ….
Hi,
How can we display current user’s name or username?
Bro you have to create a cookie for a current user
Hi Javed,
got this error on index.php page:
[09-Feb-2018 10:18:02 UTC] PHP Warning: Cannot modify header information – headers already sent by (output started at /public_html/mini/auth.php:2) in /public_html/mini/auth.php on line 5
keep getting this error. Login page is perfect. but when i am not logged in. Index page is not redirecting to Login page.
include(“auth.php”); is created to check and redirect if user is not logged in.
I have placed this file at the top of index.php.
It is working fine, but if you are still getting issue, i would recommend to check it on PHP 5.4. If this is version issue.
session_start() must be at the top of your source, no html or other output before!
you can only send session_start() one time
better to do it this way if(session_status()!=PHP_SESSION_ACTIVE) session_start()
I’m really enjoying the theme/design of your web
site. Do you ever run into any browser compativility issues?
A couple of my blog readers have complained bout my wevsite not operating correctly in Explorer but looks great in Chrome.
Do you have any recommendations to help fix this problem?
you took everything and made it real simple to understand..thanks a lot,I would so much love to be able to communicate with you…
Dear Author!
First, thanks for the great article!!
Wouldn’t it make sense to use
require instead of include auth.php??
So if for some reason, auth.php is not found/working, the script stops instead of entering wihtout authentication?
BR Tommy
I am encountering this error when logging in
mysqli_num_rows() expects parameter 1 to be mysqli_result, boolean given in E:\xampp\htdocs\final\login.php on line 24
and when i register then everything works fine but no message is displayed about successful login the screen is blank.
Thanks! this information is very good! 🙂
salam sir register is done sucessfully but login not working saying + /username/password is incorrect
same problem
same problem
Hi,
Script works, but I’m looking for something that allow me to upload files for specific users (during registration process).
Any ideas?
Thank you
When I log in it does not redirect me to the index.php it just stays on login.php why is that?
I did everything according to tutoria, however, when I put correct “login” and”password”, which ive put into database, the “Username/password is incorrect.” pops in. I cannot find where’s there’s a problem
I think the problem lays in .md5, password='”.md5($password).”‘ , but its just my guess
Congrats brother, u do a nice big work
please, check me via my email address ASAP, i have an ongoing project, but i’m stuck somehow….so i’ll appreciate to get help from you.
Javed ur Rehman bhai
migrate database mysqli to pdo pls
well unfortunately for such a clear tutorial , all the visitors comments that are facing the same issue such as i am where the login.php page only loads into blank where the connection is properly connected and username and password are correct are just being ignored.
I wish a clear answer can be provided for all of us that are not able to use this tutorial and will move on to the next google search on how to set this up
thank you for your effort ,
Kindly make sure you are using PHP 5.6 to avoid any unexpected error.
Please upload this project on github
Hi! Wouldn’t it make sense to use
require instead of include auth.php??
So if for some reason, auth.php is not found/working, the script stops instead of entering wihtout authentication?
BR Tommy
Please how can i fetch the user data from the database after login
I am already fetching user data from database, you can also look my another tutorial which link is available at the end of my tutorial.
What about forgot password?
Hi Valter,
I didn’t share any tutorial yet about it, i hope i will share it soon. Thanks
I’ve been getting the following error when trying to register:
Notice: Undefined variable: con in D:\xamp\htdocs\registration.php on line 21
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in D:\xamp\htdocs\registration.php on line 21
The lines are:
<?php
include 'db.php';
// If form submitted, insert values into the database.
if (isset($_REQUEST['username'])){
$username = stripslashes($_REQUEST['username']); // removes backslashes
$username = mysqli_real_escape_string($con,$username); //escapes special characters in a string
(No modifications to your code)
Could you help me, Javed?
As you can see it is connection error, kindly make sure that your connection is established, $con variable is defined in db.php. Please enter your database credentials here to get connected with database, such as username, password, host. Check db.php file.
Hi Javed nice eg for new in php.
In your demo page invalid error show on same page on top login form but when i try its show error login page but not showing login form. Can you help me.
Demo login is working perfectly fine, just use the detail provided for testing, i would suggest you to try this demo on different web browser.
Thank you very much, it really help me.
I am glad that you like it, share it with others 🙂
I would like to know which part of the code that hide the password during the input ? Which part of the coding change the password input into **** during login and registration form. Thank you.
That is actually html input type password, which hides password to view.
Thank you Javed. I found this very useful and easy to understand and follow through.
Thanks David, i hope you will share my tutorial in your circle.
Sir, can you please tell me where is “script” which is Pop Up if password is not entered?
I can’t find it in any file.
In login.php, there is a condition if username & password do not found it gives error otherwise it redirects to the index page.
Help a lot~
Thanks~
You welcome 🙂
My webhost moved from MySQL to MySQLi and this helped in making the transition. Thanks.
I am glad that my script help you out. 🙂
Hi how would i add it so if i wanted a status on mine and say Admin and Members options and if your an admin if shows admin and if your not it shows no link?
You can do this, all you need to do is check another check which you will create. for example create another column with name admin and INSERT 0 in that column if member is registering and 1 if Admin, normally we do not create admin through portal, we directly add admin from MySQL and enable registration option for members only.
So to view file just check if user admin value is 0 or 1. If 1 then show files.
Thanks for your tutorial. You code works fine. Keep up the good works. I added some other field to the form. I added First Name, Last Name and age. I want to fetch the whole of the data from the database from each row. This mean each data will be fetch from each column in a row. I want the result to display on index.php please what is the code i will add for this function and which page will i place the code? Thank you, God bless
To fetch records of row, you need to use this mysqli_fetch_assoc(). It needs one parameter which will be your query, you can check about it here https://www.allphptricks.com/insert-view-edit-and-delete-record-from-database-using-php-and-mysqli/
This is very usefull code thanks a lot. But i Have a problem if iam trying add a another field in registration form it shows black page please help me out from this
Make sure that you also add column in table in database.
great tutorial easy and cool thanx bro,,
You are welcome bro 🙂
hi, thanks for this tuto
i’m in local
i don’t understand something about password
when u register, u write/choose ur own password
but in the BDD, there is very complicate password… not the same i choose when i register
so :
this complicated password is sent to our email when i registered ??
or, if is not this
how can i add/replace MY own password in the BDD ??
Md5() secures the password that’s why you can’t see the real password you typed in
Adding password without encryption is not good practice, this also include the privacy of user, but if you just want to see the same password in db only for testing purpose, you can just remove md5() function from code.
Solved the issue with replacing session_start() to ob_start() and put ob_flush() before last } … like
//session_start();
ob_start();
require(‘../inc/conn.php’);
include(‘include/siteRootAdmin.php’);
and
hope this will helpful to everybody facing the header issue. 🙂
login.php not redirecting to index.php on server showing blank page, but it is working on localhost. what to do to make it work on server?
Sir thank you so much you helped me on my project,God Bless you 🙂 *hugs from Philippines.
Thanks for like it, share it with your friends 🙂
i just wanna to say thank you very much best help with us your code!!
Hello, i feel that i noticed you visited my weblog thus i came to go back the favor?.I am trying to find issues to improve my web site!I assume its adequate to use some of your ideas!!
it is not working in my additional pages.
it is working in only my home page.
for example when i opened localhost/saghar then it worked correct but when i opend localhost/saghar/contact.php it does not worked. i gave it 4/5 stars because i knew solution for it.
What is the solutions?
hi sir, thank you very much for your help/…every thing is work,but i can’t add another user,in registration…
i just wanne to say thank you very much man!!
Hi please can you help me im lost i dont understand the steps
i downloaded the demo files but when i import it to my website it loads but when i try to sign in it says cant find database how do i create database with thedemo files i downloaded
I’ve solved the issue everyone is having (after login). Here is how you fix it in 2 simple steps.
Take the Login.php code and move the HTML part in the top down to below the php code. (Note: PHP code must be in the top in order for the redirect to work). Also, add your URL into the redirect URL line: (see below).
<?php
require('db.php');
session_start();
// If form submitted, insert values into the database.
if (isset($_POST['username'])){
// removes backslashes
$username = stripslashes($_REQUEST['username']);
//escapes special characters in a string
$username = mysqli_real_escape_string($con,$username);
$password = stripslashes($_REQUEST['password']);
$password = mysqli_real_escape_string($con,$password);
//Checking is user existing in the database or not
$query = "SELECT * FROM `users` WHERE username='$username'
and password='".md5($password)."'";
$result = mysqli_query($con,$query) or die(mysql_error());
$rows = mysqli_num_rows($result);
if($rows==1){
$_SESSION['username'] = $username;
// Redirect user to index.php
header("Location: http://YOURWEBSITEURL/index.php");
}else{
echo "
Username/password is incorrect.
Click here to Login“;
}
}else{
?>
Login
Log In
Not registered yet? Register Here
Javed works great on first go! Now just gota read through the code to figure out your logic. Thx again
Hi Javed
This is brilliant. For a beginner to understand this it is purely simple and easy to understand code.
I have used everything as shown, just changed to suit my database and parameters.
The registration works perfect.
I had some problems with the login page however.
First, due to the md5 for password somehow it was reading the key stored in the database. I changed that and that is solved.
The second issue which i am unable to solve which is actually a repeat by some other comments above, is the redirect after login to the index page where it would show the username based on the session.
i havent touched your code.
$query = “SELECT * FROM `userdata` WHERE username=’$username’ and
password=’$password'”;
$result = mysqli_query($con,$query) or die(mysql_error());
$rows = mysqli_num_rows($result);
if($rows==1){
$_SESSION[‘username’] = $username;
header(“Location: index.php”); // Redirect user to index.php
}else{
echo “Username/password is incorrect.
Click here to Login“;
}
The header attribute doesnt redirect to index page to show the Welcome “username”.
It just reloads the login.php page and remains blank.
However if i manually navigate to index.php it shows be the details.
Your views please.
Thanks
Hussain
You need to push the login script to the very very top of the page, it can’t be below anything else. It’s a header…
Username/password is incorrect. even i type correct
Sir, thanks for the help, but my registration page isn’t connecting to the my database via php myadmin… Pls need urgent reply
Thank you brother, your tutorial saved my paper.It is really easy and good!
I am glad that you found it helpful, share it with others 🙂
hi Javed. this is great tutorial indeed and is simple to understand but one more problem: when do u promise to show us the reset password tutorial? apart from this, I liked your tutorial
Having an issue, mine is not working, I left my code over here: http://www.webdeveloper.com/forum/showthread.php?363723-Mysqli-issue-account-login-issue
I would be grateful if you could provide some insight!
Please help!
Notice: Undefined index: email in C:\xampp\htdocs\DASH\registration.php on line 13
You are registered successfully.
Click here to Login
Thanks for perfectly simple example, it’s exactly what I needed. Almost 🙂
Though, I want to use it only as an addition to another website, yet it insists to make user log in before they can enter main index file.
Is there a simple way to change this?
I think I figured out – if not logged in, it should redirect to homepage, and not login page.
if ( !isset( $_SESSION[ “username” ] ) ) {
header( “Location: index.php” );
exit();
}
NOPE, now I’m just getting stuck in various loops…
Hi Javed greetings thanks alot. thanks alot for your tutorial. it really helps… hope i can change the form action to the register.php in my web host?
salam sir
thanxs for this code it realy help me
thanks again 🙂
Thank you for your good work.
But after registration and login, I only get a blank page.
If I open index page when I log in, I’ll think so
BUT, you want to get to index.php directly when you log in.
This does not help
Session_start ();
Require (‘db.php’);
Hi Javed. First of all thanks for the whole tutorial…but after i successful login, it just refresh the login.php but with a totally blank…is it any php tutorial for the connection after login? and after the registration…the data that i key in was not exist in the database…
thank you i have neen using this for some time both versions . recently the <5.5 version has curiously stopped working. i cannot discover if it is a php upgrade or not as my hosting has changed. version now is 5.4.45. shame i love the simplicity of this script
I want to make sure that there is no 2 accounts have the same username
i’m getting this message when trying to log in
“Warning: session_start() [function.session-start]: Cannot send session cookie – headers already sent by (output started at C:\xampp\htdocs\xampp\seva1\login.php:9) in C:\xampp\htdocs\xampp\seva1\login.php on line 10
Warning: session_start() [function.session-start]: Cannot send session cache limiter – headers already sent (output started at C:\xampp\htdocs\xampp\seva1\login.php:9) in C:\xampp\htdocs\xampp\seva1\login.php on line 10”
Have you any video tutorial about it?
Hi am just using your registration codes, it works well but the datas username,email, password is not showing under my phpmyadmin databse.
I tried to run this on my apache server and when I navigated to the registration page all I got was a blank page. Do you know where I need to save the css file or can I just change the code to include the filepath? Also do I keep or remove all the quotations in the code?
inside the db.php file —-$con = mysqli_connect(“localhost”,”root”,””,”register”); inplace of root you should write your database name and insside “” it is for password and inplace of register you need to place your table name
I’m no expert with PHP, but I do know that md5 is totally unsafe. Shouldn’t be used for anything you care about. Aren’t there better built in PHP functions for encrypting passwords? Also, what about prepared statements SQL queries?
Hello Javed,
Thank You for posting such a wonderful blog!!!
Masha Allah…
Way to go!!!
hi, I receive the following errors while trying to register a user.
Notice: Undefined variable: con in C:\wamp64\Xamp\htdocs\Register\registration.php on line 19
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\wamp64\Xamp\htdocs\Register\registration.php on line 19
Notice: Undefined variable: con in C:\wamp64\Xamp\htdocs\Register\registration.php on line 21
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\wamp64\Xamp\htdocs\Register\registration.php on line 21
Notice: Undefined variable: con in C:\wamp64\Xamp\htdocs\Register\registration.php on line 23
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\wamp64\Xamp\htdocs\Register\registration.php on line 23
Notice: Undefined variable: con in C:\wamp64\Xamp\htdocs\Register\registration.php on line 27
Warning: mysqli_query() expects parameter 1 to be mysqli, null given in C:\wamp64\Xamp\htdocs\Register\registration.php on line 27
Exactly what I needed thank you!
Thanks Eric, if you find this helpful, i would love if you share it with friends also 🙂
thanks sir i have share it with my frinds..
Hi Javed
This warning message appear when i am opening php file on local host.
Warning: mysqli_connect(): (HY000/1049): Unknown database ‘register’ in /Applications/XAMPP/xamppfiles/htdocs/db.php on line 8
Failed to connect to MySQL: Unknown database ‘register’
What should i do next?
Thanks
Sandeep
Dear Sandeep, i would suggest you to create database with name register in your phpmyadmin, this error means it is not able to connect with DB, or you check your username or password.
WOW.
I just came by this simple registration using PHP/MYSQLI
It is lovely and a really helpful aid to newbies like me.
I really wished you could do some advanced user registration tutorial that checks for duplicate users details during registration and validation of fields.
Yes i also want to share more tutorials, unfortunately i am not able to find some time for it, because i am busy in my routine schedule.
hello
how do we add email verification to this register form
regards
Currently i just share simple user registration, yes this feature can be added, you can search on Google for now, as i didn’t write it yet.
I like your system. Can I add to the registration form a field that accepts a registration number (sent to the user in advance) that validates the number from a database list and then, if valid, continues with the rest of the registration process?
If so, can the login part of the script then give access to the private folder, or does yet another script have to exist on every html page within the private folder for each page to be accessible?
Well tom, i actually didn’t get you clearly, but if you want to give registration unique no. or id then you can simply fetch the user id and give it to user, and giving access to private folder can be possible but it will be more advance feature, and currently i didn’t write any tutorial about it.
Hi Javed, nice prog. Works OK on my Linux setup. I would suggest in order to increase password security (at least for those who use passwords in Xampp or other database setup and including phpmyadmin, to put the db.php file outside the webroot. In other words put the file in the user root in a directory called ext_include and call that in the login and registration php files as follows ‘require’/ext_include/db.php’; This means that web users cannot readily discover the phpmyadmin (database0 password.
I have altered it for my own set-up. I will when ready with other small modifications let you know to benefit all.#Well done on this, it saved me a few days headaches and paracetamols. Thanks
Yes you are right, but what i tried here, i simple explain how things work, obviously there is much things for improvement but that should be deal in the later stage, my focus was only to tell the newbie how it actually works 🙂 thanks for your input.
Awesome work my friend.Simple and easy. Respect!!!
If you find this helpful, i would love if you share it with friends also 🙂
how can I add details to my registration page I mean something like age sex state likes dislikes or for them to choose between security question and all that please how do I go about it
thanks your tutorial is awesome keep it
You need to increase field in Database, table and then take input at registration page.
when I do the copy paste of what u have instructed it works but I can’t login using the same codes in my other db, but I can register, and when I try to login after registration it shows incorrect username/password even though my username and password are correct….by the way I’ve changed db name in db.php, table name in login.php and registration.php..where should I correct more…plis help!!! presentation nearby 🙂
Sorry for the late reply, i was busy in some other tasks, well i think there may be some issue related to DB connection, you should try to connect db first, if you are changing it, and make sure if you register then its data is in database.
Hi Mr. Javad the code is not complete please I want you to send the code the complete codes of all the attributes in a PDF format into my email please thanks… daniel@**** and may I ask a question…
Do you know how to design/program a website confirmation like mmm or any ponzi scheme that when any one registers it send activation to some other user?
Dear Danny, the code is complete according to the title of web page, this is simple user registration and currently i didn’t write any tutorial which send activation code.
Goodevening Javed, thanks for the script. I am creating a volunteer website. Assuming I have 10 volunteers who have registered for the program (user-id 1-10), how can I echo the details of user-id 5 (name, phone number and department) to show on the dashboard of user-id 3 and vice-versa. Thanks.
I would suggest yo to check out my this tutorial which is part 2 of this tutorial, here you can see how to fetch data and display:
http://www.allphptricks.com/insert-view-edit-and-delete-record-from-database-using-php-and-mysqli/
Very good tutorial!
But I have problem:
“Warning: session_start(): Cannot send session cookie – headers already sent by (output started at /auth.php:8) in /auth.php on line 9”
Each file is saved without BOM (UTF-8 BOM). Only UTF-8. What can I do?
I will suggest you to start session before include DB in login page.
session_start();
require(‘db.php’);
Thank You soooooo much for sharing this! It almost saved my life. Thanks
If you found it helpful then share it with others 🙂
I used code in your download but i kept seeing this?
Warning: session_start() [function.session-start]: Cannot send session cookie – headers already sent by (output started at C:\xampp\htdocs\simple-user-registration-login\auth.php:8) in C:\xampp\htdocs\simple-user-registration-login\auth.php on line 9
Warning: session_start() [function.session-start]: Cannot send session cache limiter – headers already sent (output started at C:\xampp\htdocs\simple-user-registration-login\auth.php:8) in C:\xampp\htdocs\simple-user-registration-login\auth.php on line 9
Warning: Cannot modify header information – headers already sent by (output started at C:\xampp\htdocs\simple-user-registration-login\auth.php:8) in C:\xampp\htdocs\simple-user-registration-login\auth.php on line 11
I will suggest you to start session before include DB in login page.
session_start();
require(‘db.php’);
hi, how to store 2 usertype in php?
Need more explanation, do you want to give some specific rights to some user?
hiiii javed sir i’m very worried about my final year project if you have Online Shopping portel code please send me on my gmail account…
viju….
Well i do not have any such script right now, i didn’t write yet, but you can find it on Google, there are online e-commerce CMS available for online shopping.
I like your code and it seems it will work well. I am having an issue on all pages like registration and login, where when i fill in the information and click on the button, it just reloads the page. No error, no successful message and the database is not updated. I can use INSERT INTO and it will insert into the database so I know it is connected, but it will not do it when i use the register button
Hi Randy, i think you should first check that is your database is connected or not?
Just create a simple file of DB connection and echo connected if DB is connected else show error.
Every time I login, it just refreshes the login page, why is this? Thanks
I did not placed the redirection on login page, if you want then you can put redirection after logged in.
Javed; Great Work!
Any way to add:
1) That the member registered account expired one year after created?
2) Registration Code field – a registration code that we will print and provide to the users and when they register need to put on the registration form to validate their account.
Thanks in advance!
Thanks Samuel,
Well yes you can create expire date field and enter value in it using date() function, you can enter any date you want, just Google it and you will have lots of date formats along with future dates.
and for registration code i actually didn’t get you clearly but if you are saying that user will provide registration code which is provide to them manually, then you can simply compare any field using any value either in database or in PHP page if registration code is similar. Otherwise you will need to create another table in DB add all the Reg. Code there and find the user provided code in the table first, if it matches then proceed to register.
Hi Javed,
When I try to register it gives me this error msg: “Failed to connect to MySQL: Access denied for user ‘root’@’localhost’ (using password: NO)”
,so what can I do to fix that??
Thanks in advance
Kindly check is your provided password is same as your localhost phpmyadmin?
thanks javed man,it finally work i really appreciate this Rasaq from nigeria
I am glad that you found it helpful.
hi, i cant figure why after i try success register, when i try to login it either give me error message username/password incorrect and blank page, wasnt it suppose to go to index.php? help me.
Try using user and password without space.
I have used the code in the download file that you provided. I keep getting this when I login:
Warning: session_start(): Cannot send session cache limiter – headers already sent (output started at /public_html/login.php:9) in /home/s1541/public_html/login.php on line 11
Warning: Cannot modify header information – headers already sent by (output started at /home/s1541/public_html/login.php:9) in /home/s1541/public_html/login.php on line 28
I will suggest you to start session before include DB in login page.
session_start();
require(“db.php”);
Hi Javed,
Thank you for this simple script and i was able to learn much, but i would like to ask about this part
require(‘db.php’);
if (isset($_REQUEST[‘username’])){
$username = stripslashes($_REQUEST[‘username’]);
$username = mysqli_real_escape_string($con,$username);
the username on the if line, whenever i change it the script would stop working/not redirect to any page and i would like to know how does it work or if it does grab something from the db.php, thank you
As you can see that form is submitting on the same page, i am checking here that if this field is filled, which means that form is submitted then perform the actions mention in the parenthesis {}.
thanks bro
i made with your support
stil i need to learn php advance can you tell me how to learn it
Well i suggest you to start working with an organization so that they can assign you task, you will learn more when you will have tasks. You can search your queries on Google.
Hi Javed,
This script is simple and straight to the point. Thank you.
Everything works wonderfully on my site, however, I am having one issue where after login the login.php does not redirect to index.php. So after a successful login, the page refreshes login.php and it is blank. If I manually go to the index.php the session is open and the users info will display correctly. Can you help with the redirect on login to index?
Try to register with plain user and password, like
username: user
password: pass
If your issue is resolved then just simply check mysqli_real_escape_string() function because it escape special characters in variables.
This helped me out! Thanks!
One small thing I’ve added is an link back from register to login, for users that didn’t want to register but expediently clicked on it.
Thanks!
Great addition.
bare in mind i am using online hosting but not local server
Hello javed
Thank you for sharing this post.
It worked fine till registration and login but once i registered my self and use the login and password which i have created using registration page it doesn’t redirect to index page but gives this error
Cannot modify header information – headers already sent by (****/****/public_html/myaccount/register/login.php:11) in /****/****/public_html/myaccount/register/login.php on line 28
This kind of error occurs when you echo or print something before header redirection. Check your code is something printing before redirect.
I am getting the same message when I try to login.
………….. Code is Skipped
This is the message that i keep getting after logging in:
Warning: Cannot modify header information – headers already sent by (output started at /home/soiree/public_html/Login/login.php:43) in /home/soiree/public_html/Login/login.php on line 60
I will suggest you to start session before include DB in login page.
session_start();
require(‘db.php’);
Hi Javed, first i would like to tell thanks to you!! The code is going fine upto login page when i enter correct username and password it shows incorrect username or password.
Hi Shamanth thanks that you find it helpful, if you are talking about demo link then i have place there demo user and password, you can login only with that one, or if you have set it up on your local server then you should check after registration that is your registration is going into database? If not then you should enable your local phpmyadmin. Or you can try it online if you have any hosting available.
This is exactly what I needed for my existing website. I’m smart enough to figure how to add more required fields and insert the info to my db. However, is there an easy way for me to display a username on a page? As in “Hi [username], welcome to my site”.
Yes there is a way, just write a query to fetch username from database and print it.
Dear Mr. Javed,
Thank you for your good work. I need a full payment system script for my small business where my customers can login and make and receive payments. Is this possible
Dear Mark, i am glad that you like it, but for payment system there are various options it depends that which option you want to use, like PayPal, or any other payment method. Script for payment will be vary according to your and payment service provider needs.
Great tutorial! However, it worked fine for me at first but now when I try to register a blank page just appears. Any ideas?
It seems like after clicking on register button you are getting error, although you can see in my code that if form is submitted it must show either error or successful message, if you can explain it in more detail so may i can i figure out what is actually issue you are facing, are you doing it local server or on host? try it host if you are facing issue in local server, may be problem in your local server or not configured properly.
Cheers for the reply. When i attempt to register a user the error or successful message doesn’t load, the page just appears blank. It’s on host just now and I still can’t figure out the error
Try to register with plain user and password, like
username: user
password: pass
If your issue is resolved then just simply check mysqli_real_escape_string() function because it escape special characters in variables.
Thanks, Helped a lot.
How about if I want to check if the username already exists in the database?
Yes you can add this, all you need is to check in the same table if username already exist or not, if exist show error else insert record.
Hello Javed. Your tutorial and code is awesome. pls can you help implement the query that checks during sign up if a user already exists in the database table; and if it does, the form should display an error message. Thank you so much.
Mr Javed good work, but I keep getting a connection timed out error
Dear Bob, usually this error because of windows issue, you may check this to solve issue.
http://www.incrediblelab.com/connection-timed-out-error-fixed/
http://windowsreport.com/connection-timed-out-windows-10/
What about Reset Password and Change Password???
Currently i just created simple registration form, i will try to wrote tutorial about it also in coming future.
This is very handy! thank you Javed!
Glad to see that you like it. 🙂
I have tried to make it work but any page that calls the auth.php file seems to just output the code onto the page and not show what it should.
Can you please tell me what error it is actually showing to you. Auth file is only to check is user logged in or not.
Mr. Javed good day I am Isah from Nigeria I have experienced difficulties in running your code on notepad++ please send the way out through ******
Dear Isah, can you please tell me what kind of problem you are facing? it does not matter what IDE you use, we use IDE to just write and edit our code, if you do not have idea how to run php files on localhost then you can check my tutorial about it. http://www.allphptricks.com/how-to-run-php-files-on-localhost/
This is Wright Ian, The code works if you always used to mysqli instead of MySQL….. Try it again it works….
Hi there this really help me a lot. How can i implement a function that check user if exist in registration form??
Hi you will need to check in database if user is already registered with same email then you can display a message that user already exist.
Thanks so much. Helped me today!
I’m glad to see that you found it helpful 🙂
I have got these 2 warnings, please help.
Warning: mysqli_select_db() expects exactly 2 parameters, 1 given in C:\wamp64\www\fresh\db.php on line 6
&
Warning: mysqli_error() expects exactly 1 parameter, 0 given in C:\wamp64\www\fresh\db.php on line 8
Please help!
Thank you.
If you are using mysqli_select_db() then you need to provide connection also in your parameter.
The mysql_select_db() does not work in MySQL Improved, Try adding the database as fourth parameter….
For example, if I’m connecting to a Db named Wright, I’ll write,
Mysqli_connect_db(“localhost”,”root”,”password”,”Wright”)
This works every time for MYSQLI
This is the correct code Mr.Khalfay
Mysqli_connect_db(“localhost”,”root”,”password”,”Wright”)
Hello!
I followed the directions here exactly!
But I can’t seem to get this to connect to my database, I keep getting the code
“Database Selection FailedAccess denied for user ‘mikereve’@’localhost’ (using password: NO)”
At the top line of my login page.
Please advise! 🙂
You need to make sure that you are providing the correct detail for connecting database, commonly root is the user and make sure you are providing the correct password, if there is no password in your phpmyadmin so you do not need to provide password on local environment.
Your post is just awesome sir
Thanks u so much and your one is just awesome
Very useful thanks….
In your registration form, what is the mechanism of user already exists in the database. The same user can be inserted any time. plz check it
Thanks for stopping by, Naveed yes you are right but i didn’t use here to check is user already exist or not, i tried to explain simple registration for newbie users. Yes we can implement so many things in registration form. 🙂
Thanks Javed Ur Rehman Sir Its helps me alot….
Thanks glad that you like it. 🙂