Insert, View, Edit and Delete Record from Database Using PHP and MySQLi
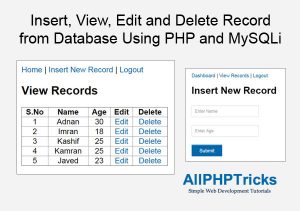
In this tutorial, I will explain how to insert, view, edit and delete record from database using PHP and Mysqli, basically this tutorial is a second part of Simple User Registration & Login Script in PHP and MySQLi, in this first part I explained how to create simple user registration and login using PHP and MySQLi, if you do not know how to create user registration and user login form so kindly first check this tutorial Simple User Registration & Login Script in PHP and MySQLi, after that now come back to this tutorial.
I have tested this tutorial code from PHP 5.6 to PHP 8.2 versions, it is working smoothly on all PHP versions. However, I would recommend to use the latest PHP version.
I would suggest you to check PHP CRUD Operations Using PDO Prepared Statements, as this is compatible with PHP 7 and PHP 8 both versions.
Steps to Creating an Insert, View, Edit and Delete Record from Database Using PHP and MySQLi
Now I am assuming that you have already created user registration and login forms which I created in Simple User Registration & Login Script in PHP and MySQLi now I will create another table to keeping records in it, update dashboard.php file and add four more new pages which are insert.php, view.php, edit.php, and delete.php, follow the following steps:
- Create Another Table for Records
- Update Dashboard File
- Create Insert Page
- Create View Page
- Create Edit/Update Page
- Create Delete Page
1. Create Another Table for Records
Execute the below SQL query:
CREATE TABLE IF NOT EXISTS `new_record` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`trn_date` datetime NOT NULL,
`name` varchar(50) NOT NULL,
`age`int(11) NOT NULL,
`submittedby` varchar(50) NOT NULL,
PRIMARY KEY (`id`)
);
2. Update Dashboard Page
Update dashboard.php file and paste the following code in it.
<?php
include("auth.php");
require('db.php');
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Dashboard - Secured Page</title>
<link rel="stylesheet" href="css/style.css" />
</head>
<body>
<div class="form">
<p>Welcome to Dashboard.</p>
<p><a href="index.php">Home</a><p>
<p><a href="insert.php">Insert New Record</a></p>
<p><a href="view.php">View Records</a><p>
<p><a href="logout.php">Logout</a></p>
</div>
</body>
</html>
3. Create Insert Page
Create a page with name insert.php and paste the below code in it.
<?php
include("auth.php");
require('db.php');
$status = "";
if(isset($_POST['new']) && $_POST['new']==1){
$trn_date = date("Y-m-d H:i:s");
$name =$_REQUEST['name'];
$age = $_REQUEST['age'];
$submittedby = $_SESSION["username"];
$ins_query="insert into new_record
(`trn_date`,`name`,`age`,`submittedby`)values
('$trn_date','$name','$age','$submittedby')";
mysqli_query($con,$ins_query)
or die(mysqli_error($con));
$status = "New Record Inserted Successfully.
</br></br><a href='view.php'>View Inserted Record</a>";
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Insert New Record</title>
<link rel="stylesheet" href="css/style.css" />
</head>
<body>
<div class="form">
<p><a href="dashboard.php">Dashboard</a>
| <a href="view.php">View Records</a>
| <a href="logout.php">Logout</a></p>
<div>
<h1>Insert New Record</h1>
<form name="form" method="post" action="">
<input type="hidden" name="new" value="1" />
<p><input type="text" name="name" placeholder="Enter Name" required /></p>
<p><input type="text" name="age" placeholder="Enter Age" required /></p>
<p><input name="submit" type="submit" value="Submit" /></p>
</form>
<p style="color:#FF0000;"><?php echo $status; ?></p>
</div>
</div>
</body>
</html>
Readers Also Read: Laravel 10 CRUD Application
4. Create View Page
Create a page with name view.php and paste the below code in it.
<?php
include("auth.php");
require('db.php');
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>View Records</title>
<link rel="stylesheet" href="css/style.css" />
</head>
<body>
<div class="form">
<p><a href="index.php">Home</a>
| <a href="insert.php">Insert New Record</a>
| <a href="logout.php">Logout</a></p>
<h2>View Records</h2>
<table width="100%" border="1" style="border-collapse:collapse;">
<thead>
<tr>
<th><strong>S.No</strong></th>
<th><strong>Name</strong></th>
<th><strong>Age</strong></th>
<th><strong>Edit</strong></th>
<th><strong>Delete</strong></th>
</tr>
</thead>
<tbody>
<?php
$count=1;
$sel_query="Select * from new_record ORDER BY id desc;";
$result = mysqli_query($con,$sel_query);
while($row = mysqli_fetch_assoc($result)) { ?>
<tr><td align="center"><?php echo $count; ?></td>
<td align="center"><?php echo $row["name"]; ?></td>
<td align="center"><?php echo $row["age"]; ?></td>
<td align="center">
<a href="edit.php?id=<?php echo $row["id"]; ?>">Edit</a>
</td>
<td align="center">
<a href="delete.php?id=<?php echo $row["id"]; ?>">Delete</a>
</td>
</tr>
<?php $count++; } ?>
</tbody>
</table>
</div>
</body>
</html>
Readers Also Read: Laravel 10 User Roles and Permissions
5. Create Edit/Update Page
Create a page with name edit.php and paste the below code in it.
<?php
include("auth.php");
require('db.php');
$id=$_REQUEST['id'];
$query = "SELECT * from new_record where id='".$id."'";
$result = mysqli_query($con, $query) or die ( mysqli_error($con));
$row = mysqli_fetch_assoc($result);
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Update Record</title>
<link rel="stylesheet" href="css/style.css" />
</head>
<body>
<div class="form">
<p><a href="dashboard.php">Dashboard</a>
| <a href="insert.php">Insert New Record</a>
| <a href="logout.php">Logout</a></p>
<h1>Update Record</h1>
<?php
$status = "";
if(isset($_POST['new']) && $_POST['new']==1)
{
$id=$_REQUEST['id'];
$trn_date = date("Y-m-d H:i:s");
$name =$_REQUEST['name'];
$age =$_REQUEST['age'];
$submittedby = $_SESSION["username"];
$update="update new_record set trn_date='".$trn_date."',
name='".$name."', age='".$age."',
submittedby='".$submittedby."' where id='".$id."'";
mysqli_query($con, $update) or die(mysqli_error($con));
$status = "Record Updated Successfully. </br></br>
<a href='view.php'>View Updated Record</a>";
echo '<p style="color:#FF0000;">'.$status.'</p>';
}else {
?>
<div>
<form name="form" method="post" action="">
<input type="hidden" name="new" value="1" />
<input name="id" type="hidden" value="<?php echo $row['id'];?>" />
<p><input type="text" name="name" placeholder="Enter Name"
required value="<?php echo $row['name'];?>" /></p>
<p><input type="text" name="age" placeholder="Enter Age"
required value="<?php echo $row['age'];?>" /></p>
<p><input name="submit" type="submit" value="Update" /></p>
</form>
<?php } ?>
</div>
</div>
</body>
</html>
6. Create Delete Page
Create a page with name delete.php and paste the below code in it.
<?php
require('db.php');
$id=$_REQUEST['id'];
$query = "DELETE FROM new_record WHERE id=$id";
$result = mysqli_query($con,$query) or die ( mysqli_error($con));
header("Location: view.php");
exit();
?>
Click here to learn how to implement forgot password recovery (reset) using PHP and MySQL.
This tutorial is only to explain the basic concept of PHP CRUD operations, you will need to implement more security when working with live or production environment.
If you found this tutorial helpful so share it with your friends, developer groups and leave your comment.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks
Please update this to php7, its been many years and out dated.
Sure, I will soon share the updated tutorial with latest version. Thanks for the suggestion.
Thanks, it is working perfectly.
This code is vulnerable to SQL injection attack.
Dear Azeem,
The purpose of this tutorial is to explain the simplest flow, indeed there is a lot of things to do to validate the data but this will create complexity for novice user.
it’s perfect dear and very helpful
Warning: mysqli_num_rows() expects parameter 1 to be mysqli_result, bool given in D:\New folder\htdocs\Welcome\Admin_login.php on line 61
actual error is on
Notice: Undefined index: job_provider_id in C:\……edit_provider.php on line 3
Dear Javed Sir,
the error :Undefined index: job_provider_id show in my code please please help
Update Record
Update Record
<?php
$status = "";
if(isset($_POST['new']) && $_POST['new']==1)
{
$name =$_REQUEST['job_provider_name'];
$email =$_REQUEST['job_provider_email'];
$pass = $_REQUEST['job_provider_pass'];
$cmp_name = $_REQUEST['job_provider_cmp_name'];
$address = $_REQUEST['job_provider_address'];
$phone = $_REQUEST['job_provider_phone'];
$update="update job_provider set
job_provider_name='".$name."', job_provider_email='".$email."',
job_provider_pass='".$pass."', job_provider_cmp_name='".$cmp_name."', job_provider_address='".$address."', job_provider_phone='".$phone."' where id='".$id."'";
mysqli_query($con, $update) or die(mysqli_error());
$status = "Record Updated Successfully.
View Updated Record“;
echo ”.$status.”;
}else {
?>
<input name="id" type="hidden" value="” />
<input type="text" name="job_provider_name" placeholder="Enter Name"
required value="” />
<input type="text" name="job_provider_email" placeholder="Enter "
required value="” />
<input type="text" name="job_provider_pass" placeholder="Enter "
required value="” />
<input type="text" name="job_provider_cmp_name" placeholder="Enter "
required value="” />
<input type="text" name="job_provider_address" placeholder="Enter "
required value="” />
<input type="text" name="job_provider_phone" placeholder="Enter "
required value="” />
I think you should provide id value in this field.
The error you are getting is may be because of your database table column name.
In order to fix your error, I will need to see your code as your query is not about my tutorial, it is something else.
How to View Records where submittedby = $_SESSION[“username”];
In the view page, you can query result based on submittedby = $_SESSION[“username”]; And it will display the desired result.
Hi Javed sir, thanks for sharing the very best blog for me because I learn PHP web development and this blog is very useful and helpful for me.
Thanks again.
You welcome, thanks for the appreciation.
hi can you help me in my task
Hi Javed first of all thank you so much for this nice script !!
I did created the database and installed all files on my directory. I did register myself and it actually created my account in the users table.
BUT when I login, the page go blank (white) no error message but wont go further…
If I try to go straith to index.php… same think page stay blank white
Same with dashboard.
If I enter wrong credentials then I get a proper Wrong Username/password message..
Do you know what could be the problem ?
move the session_start on the top of the page.
Move it to?
Top of the page means first thing should be session_start in the page. Hope this help you.
HI Javed can we do edit and insert in same form? with out using multiple forms.
Yes it is possible, I created separate pages to keep them simple as possible, you can combine them all, but this will change the entire logic of application.
Hi, I just want to ask on how to distinguish the admin function and user function. Thank you
Basically, this tutorial is focused on how to use the CRUD operation, well you can store value into column, for example for admin store 1 and for others store 2 in the user_type column.
Very well written and done.
I’ve juet started writing in the last few days and have seen loot of articles
simply rework old ideas bbut add vrry little of worth. It’s great to see an informative post of some true value to your
readers and me.
It is on the list of creteria I need to replicate as a
new blogger. Reeader engagement annd material value arre king.
Many supeerb thoughts; you’ve certainly mahaged to get on my list of sites to
follow!
Continue the terrific work!
Cheers,
Stanislas
Thanks for the appreciation.
Thank you for your great article. It is very helpful and easy to understand coding.
Keep it on Boss!
Thanks for the appreciation.
Please post an article for:
1. CRUD with live search, Pagination, Delete Confirmation dialogue.
2. mpdf for Indic Complex Unicode Language
3. Table with listing records in php using MySql Query: Group by & Order by
@Javed what might be wrong here
Warning: mysqli_fetch_assoc() expects parameter 1 to be mysqli_result, boolean given in C:\wamp64\www\hospital-management\edits.php on line 6
Call Stack
# Time Memory Function Location
1 0.0015 245616 {main}( ) …\edits.php:0
2 0.0864 255816 mysqli_fetch_assoc ( ) …\edits.php:6
Update Record
Update Record
<?php
$status = "";
if (isset($_POST['new']) && $_POST['new'] == 1) {
$id = $_REQUEST['id'];
$name = $_REQUEST['nur_name'];
$phone = $_REQUEST['nur_phone'];
$quali = $_SESSION["nur_quali"];
$update = "update nurse set nur_name='" . $name . "',
nur_phone='" . $phone . "', quali='" . $quali . "',
where id='" . $id . "'";
mysqli_query($con, $update);
$status = "Record Updated Successfully.
View Updated Record“;
echo ” . $status . ”;
} else {
?>
<input name="id" type="hidden" value="” />
<input type="text" name="nur_name" placeholder="Enter Name" required value="” />
<input type="text" name="nur_phone" placeholder="Enter Age" required value="” />
<input type="text" name="nur_quali" placeholder="Enter Age" required value="” />
You can not place comma (,) before WHERE clause, kindly check this line and remove comma before WHERE.
quali='” . $quali . “‘, where
Amazing tutorial but one things not worked for me. You added ‘submittedby’ method but when I logged in from another account data shows data inserted by previous user. Any solution for this please?
When user logged in, it store the username in session $_SESSION[“username”], you can simply print this value on any PHP, it does not matter and check if its value is changing after login with different users. It should be change. Hope this will solve your issue.
Warning: mysqli_prepare() expects parameter 1 to be mysqli, null given in C:\wamp\www\insert-view-edit-and-delete-record\registration.php on line 21
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\wamp\www\insert-view-edit-and-delete-record\registration.php on line 23
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\wamp\www\insert-view-edit-and-delete-record\registration.php on line 25
Warning: mysqli_query() expects parameter 1 to be mysqli, null given in C:\wamp\www\insert-view-edit-and-delete-record\registration.php on line 29
Can you explain to me what ‘submittedby’ used for?
It will help you later that who actually submitted this data.
When I refresh the page, it keeps adding the same record I already did. The more i refresh, the more it inserts. How to stop that when people refresh?
Just check if record is already exist by user then show the error message and if there is no record found, insert the data in table. Hope this help you.
Add this script just before body closing tag.
if ( window.history.replaceState ) {
window.history.replaceState( null, null, window.location.href );
}
What is included in db.php?
Your database connection.
Please help me
Kindly share your problem in detail.
Thank you very much for your work.
i`m gettint eror message on …
Fatal error: Uncaught Error: Call to undefined function mysql_error() in C:\xampp\htdocs\subscription\insert.php:14 Stack trace: #0 {main} thrown in C:\xampp\htdocs\subscription\insert.php on line 1
than
i changed the msql to msqli but now the error change to…
Warning: mysqli_error() expects exactly 1 parameter, 0 given in C:\xampp\htdocs\subscription\insert.php on line 14
what do you recommend please?
Pass the connection in mysqli_error($con)
sir, i am natarajan, which php developer is best for developing the forms and report , i need master details report and tab function form ,it is possible to develop the php,
You can develop almost all kinds of forms and reports using PHP and other languages such as HTML, CSS, JavaScipt, jQuery, and AJAX.
Well that’s helpful thanks for the share, and keep up the good work.
Thanks for simple code sir .i have one issue that is in view data viewed in descending order . i also removed the desc in sql query . please reply me.
Just replace desc to ASC to view data in Ascending order.
Hi sir.. I want to show a single record details from database. When i click on this record.
Yes it is possible to display single record. Unfortunately, I didn’t publish any such tutorial but you can find it here https://www.w3schools.com/php/php_mysql_select_where.asp
Hi Javed thank, can you help me this errors for login
Connected successfully
Notice: Undefined variable: con in C:\xampp\htdocs\keys\login.php on line 17
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\xampp\htdocs\keys\login.php on line 17
Notice: Undefined variable: con in C:\xampp\htdocs\keys\login.php on line 19
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\xampp\htdocs\keys\login.php on line 19
Notice: Undefined variable: con in C:\xampp\htdocs\keys\login.php on line 23
Warning: mysqli_query() expects parameter 1 to be mysqli, null given in C:\xampp\htdocs\keys\login.php on line 23
Fatal error: Uncaught Error: Call to undefined function mysql_error() in C:\xampp\htdocs\keys\login.php:23 Stack trace: #0 {main} thrown in C:\xampp\htdocs\keys\login.php on line 23
Hi Salma, Seems like you are not able to connect to the database.
Also kindly change mysql into mysqli, if you found any.
hi, javed i am not able to connect to the database
i change mysqi into mysql and i get this errors
Fatal error: Uncaught Error: Call to undefined function mysql_connect() in C:\xampp\htdocs\keys\db.php:7 Stack trace: #0 C:\xampp\htdocs\keys\login.php(10): require() #1 {main} thrown in C:\xampp\htdocs\keys\db.php on line 7
hi javed, thank you trying to help me but i am still unable to connect to the dababase i mention before and i don’t know what i missing
and i get this message from localhost
Failed to connect to MySQL
and i changed mysqli into mysql
didnot show me any thing
thank
You welcome Salma, But i said to change mysql into mysqli, not mysqli into mysql.
You can also check database connection from W3schools as well.
hi javed can you help me i am not able to connect to the database
You can check my simple user registration tutorial for database connection.
I downloaded the tutorial and ran it and it works as planned! However, when I switch to another table I can get data to display and can delete a record but when I try to edit a field it does not work. I have checked that the fields have same text-encoding, unsigned, type, length etc, but still cannot get it to work. I have also checked variable and field names for error and there are none. Any help would be appreciated.
I have already fixed your issue, i hope you found it helpful.
Please I have an issue updating a mysql database table from my webpage using php, I will appreciate any help rendered. The data were fetch and display in an HTML table.
I would suggest you first check if your database connection is working or not. Kindly test my demo first, if you are still not able to update then you will need to debug the code manually, try to connect database and print values for testing, and check if it is connected or not, then insert value into database. If insertion is working then updating will work too.
I love this , so easy to comprehend. Thank you so much.
Thanks for this tutorial it helps me allot
I want to count rows.
How do you edit a page on the that is existing on the site.
Thanks for the coding and tutorial, but when i applied it, it always getting an error… {“code”:”MethodNotAllowedError”,”message”:”POST is not allowed”}. Do you know why it appeared? thank you
Contact your hosting provider to make sure that POST method is not disabled there.
Hi, did u inserted the more inputs.i have tried but i am getting undefined index.please help me with this.
thank you, everything is very well. it is very helpful.
You welcome and thanks for the appreciation.
You are a super man
i marked full web database following you
thank you very much
Thank you so much for creating this website. I was able to create a my own system with PHP and MySQL because of your generosity to share your knowledge through your beautiful website. Can you please create a tutorial about “Comments & Reply System”, “Ratings (like giving likes, dislikes, or stars)”, and “basic Facebook-like app with messaging, posting, adding or removing friend features, and or follow or unfollow”?
Dear Jonas,
Thanks for the appreciation, well sure i will create more tutorials as soon as i get some spare time.
Thanks for these scripts, they help a lot.
I am having an issue with trying to edit data from joined tables.
I can get to the “view records” page fine but am having trouble trying to figure out how to edit data.
I have two tables joined by an ID Number. I would like, when I click edit, to be able to see and edit data from both tables on the single edit screen. I can add fields from T1 fine, but when I try to add fields from T2 I get an undefined index error. I do not know how to get it to pull data from the second table and then update that data.
As’Salam Alikum,
very good tutorial thanks for sharing. I have tried it and i am wondering if its possible to create users with privileges to not view other users data so that one user can’t see another user’s data. if you have any idea than please share it.
thanks
Walaikum As’Salam,
Yes Ghani, it is possible but you will need to make changes accordingly.
Hello. I have been looking for edit and update page examples online that let you select which table in a database to work on. It seems all examples I find are limited to working on just one table in the database. Is it possible to select a table to edit? That would be a great tutorial.
Warning: Cannot modify header information – headers already sent by (output started at C:\AppServ\www\insert-view-edit-and-delete-record\auth.php:8) in C:\AppServ\www\insert-view-edit-and-delete-record\auth.php on line 11
Something is already printed. Look into the code what is printing before redirect.
it says Mailer Error: SMTP connect() failed. https://github.com/PHPMailer/PHPMailer/wiki/Troubleshooting
Make sure your credentials are correct.
It’s giving me unexpected if
Kindly mention clearly, where are you getting this error.
if we want to amend or change a paritcular or some particular field instead of whole record or change/delete whole record
Yes everything is possible but you will need to create another page for these actions. Or use ajax method to update fields individually.
Brother man.Thanks please email me back
I’d be interested to see a dynamic field add in the form, and how you handle the array submitted to the insert page. Can you show that?
I am very interested with your tutorial may Allah help you and guide everywhere you are. Thanks a lot.
Thanks bro for the appreciation.
I have a question. You use md5, but how can I use password_hash in login and registration?
Hi!
I get this error message when I log in
Warning: mysqli_error() expects exactly 1 parameter, 0 given in /home/public_html/insert/login.php on line 28
This works great! However how would I automatically select the correct value in a dropdown when editing a record?
Hello Javed,
I’m trying to edit the table with ajax, and I’m using $wpdb wordpress hook, can you please tell me what would be the code to edit the table from backend, my table columns are : “links” and “hitstocount”
So when I want to edit any particular link I’ll need to add a button in front of each of these rows?
What would be the exact code to do so?
Thank you!
WordPress have its own structure, you need to follow it, learn how to use ajax in WordPress, you will need to either create a plugin or work on themes function.php file. Unfortunately, currently i didn’t share any tutorial about it, but you can find it easily.
Hi,its a very great coding.
but may i know why u use “$count=1;” and end with “$count++;” ?
I need to know because i still new in programming.
Thank you
It is used to display serial number on first column.
I need help.
I have one page , where i put registration page with firstname, lastname,age,group.. and in same page below
registration page there is show data all data coming from data base.. now i have to put edit button on each row of show data.. when i click edit button ,, the whole row get blank and two more button come cancel button and update button.. on same row..then when i put updated data on that blank row , then i click update.. please send these code.
Hi Sanjeet,
I have already shared how to insert, edit, view and delete in very simple way using PHP.
I would suggest, first download my tutorial and try to execute the same file. If it is working then understand how the codes are working through my tutorial explanation then try to edit code as per your need. Hope this will help you to edit.
Hi i have problem
Undefined variable: status in C:\xmapp\htdocs\___\action.php on line 10
and this bro please help me…
Undefined index: id in C:\xmapp\htdocs\travels\action.php on line 4
i already defined but system throw tis error.
what auth.php program
contains???
It checks that user is logged in or not.
Good night, what if I want to insert, update (edit) and delete a specific row in the table without switching page? Per example, I click “edit” and a pop up appears where I can modify the data… Or I click “insert” and a pop up appears where i can insert a new row… and the same for deleting…
Thank you!
Yes it is possible, but you will need to write different code for it and use ajax to update database.
It is very helpful Thank you for your help
But in my case insert.php is not working line 12 $stn_date(“Y-m-d H:i:s”) is not responde to me .
It says pares error.
In my code in insert.php there is nothing like you said “$stn_date(“Y-m-d H:i:s”)”
How can delete data but first asked if you’re sure to delete or not?
Yes i agree, you can confirm JavaScript confirm function, i made this simple therefore i didn’t use it but you can use.
Hi I encounter this error.
Fatal error: Uncaught Error: Call to undefined function mysql_error() in C:\xampp\htdocs\impex insert.php:19 Stack trace: #0 {main} thrown in C:\xampp\htdocs\impex insert.php on line 19
use mysqli_error() function instead mysql_error() because in lastest php version is not supported mysql_error() function
Thank You. It is very simple to learn.
Thank You.
Hi good job,please add picture and validation to this tutorial
Hi Selma,
I have already created some tutorial which can help you to upload picture with validation. Check following tutorials:
https://www.allphptricks.com/upload-file-using-php-save-directory/
https://www.allphptricks.com/check-file-size-extension-uploading-using-jquery/
Thanks for this clear and simple code, I was lookin for this, tons of videos or examples didn’t work, this one does! Everything works fine!
Only a suggestion: On the view.php, when click on delete, would be great to before the entry is deleted, a popup/alert/warning to ask if really want to delete it.
Hi Rock Rubio,
Thanks for the appreciation, yes you are right, on this stage i tried to make it simple as possible, yes there are lot of validation that should be added.
You can apply confirm() to perform your desired action. You can learn about it here https://www.w3schools.com/jsref/met_win_confirm.asp
I want to add a button in the place of age in table when the user clicks the download button the file should download which is uploaded when admin is inserting data
ex:-
name:-something
age:-22
I want
name:-something
Download link (instead of showing age i want a button which user can download file ).
Warning: session_start() [function.session-start]: Cannot send session cache limiter – headers already sent (output started at C:\xampp\htdocs\insert-view-edit-and-delete-record\login.php:15) in C:\xampp\htdocs\insert-view-edit-and-delete-record\login.php on line 16
Warning: Cannot modify header information – headers already sent by (output started at C:\xampp\htdocs\insert-view-edit-and-delete-record\login.php:15) in C:\xampp\htdocs\insert-view-edit-and-delete-record\login.php on line 33
sir i have errors like that at the beginnig plz tell me what to do?
Move session_start() function at the beginning of each page.
Sir please give your contact number
Dear Naresh,
If you have any query related to the tutorial, leave it here in the comment section, or if you want to hire me, send me email here [email protected]
Q: What is you prefer? Programming or Technical?
A: Yes.
Hi, thanks for the great article. Can you please add the functionality to Search database records?
Thanks i hope i will make it.
Hi bro, nice its works perfectly but in login page.
Warning: session_start(): Cannot send session cache limiter – headers already sent
can tel me whats problem?
Hi Shashi, kindly check on your all pages that session_start() function must be at the beginning of the page, if does not, kindly move it on the top of every PHP pages, this will solve your issue.
Excellent work…
Expecting many more as i m new to web development
I am glad that you liked it.
How to upload to live server after trail on xampp server.
Hi, thanks for the tutorial.
where should I make change to switch to another table? I’m trying to point to another table in the database, so I tried renaming new_record to the one I want (in insert.php), but the data is not saved to the new table.
If you are facing issue during inserting into new table, first make sure that you have created table in database and check your column names clearly, and insert very simple record in it via PHPmyAdmin and then add records from PHP.
I’ve created my new table, made all the columns the same as in new_record table, insert a simple record then add record from PHP as you stated. I still get the error
Fatal error: Uncaught Error: Call to undefined function mysql_error() in C:\xampp\htdocs\record\insert.php:37 Stack trace: #0 {main} thrown in C:\xampp\htdocs\record\insert.php on line 37
where line 37:
mysqli_query($con,$ins_query) or die (mysql_error()) ;
thanks for your help
Replace the mysql_error() with mysqli_error($con)
A big help. Where is the css/style.css?
Thanks
Download the tutorial to get the complete files. However, i have already shared all code in the tutorial of part 1 here https://www.allphptricks.com/simple-user-registration-login-script-in-php-and-mysqli/.
Please can you add category (page)?
With image update karaiye
Sir, thanks for uploading the codes. They are really useful. I am using only the ‘users’ table and performing the update, delete and view actions on it.
There are two problems that I am facing.
Number One: when inserting new user in the table, it is working perfectly. But if I logout and go to the login page and enter that same username & password added recently, it says invalid username/password.
Number two: While performing the update, it gives error like:
Warning: mysqli_error() expects exactly 1 parameter, 0 given in C:\xampp\htdocs\NEW\edit.php on line 34….
and this is the code snippet-
if(isset($_POST[‘new’]) && $_POST[‘new’]==1)
{
$id=$_REQUEST[‘id’];
$trn_date = date(“Y-m-d H:i:s”);
$username =$_REQUEST[‘username’];
$email =$_REQUEST[’email’];
$password=$_REQUEST[‘password’];
$submittedby = $_SESSION[‘username’];
$update=”update new_record set trn_date='”.$trn_date.”‘, username='”.$username.”‘, email='”.$email.”‘, password='”.$password.”‘ , submittedby='”.$submittedby.”‘
where id='”.$id.”‘ “;
mysqli_query($con, $update) or die(mysqli_error()); <– giving the error here
$status = "Record Updated Successfully.
Hi Suman, To log in you must need to check either user is inserted in database or not, and then when you are trying to log in so try to echo value of user and password on the form submission so you can debug where there error is.
And for 2nd error, simply replace your mysqli_error(); with mysqli_error($con);
Hi Mr Javed Ur Rehman. I like your tutorial thank you.
I had the same problem and did what you told Suman.It works but it gives me a syntax error: here is the errore below
You have an error in your SQL syntax; check the manual that corresponds to your MariaDB server version for the right syntax to use near ‘s ‘, age=’23’, submittedby=’Benedictus’ where id=’19” at line 2
Seems like you are using incorrect inverted commas in ID:
id=’19” both commas should be either single or double.
sir,
where is the db.php and auth.php file?
Dear Khadija,
This is the 2nd part of tutorial, you can find db.php and auth.php in its 1st part. https://www.allphptricks.com/simple-user-registration-login-script-in-php-and-mysqli/
You can get these both files by downloading the tutorial, i have uploaded all files in tutorial download.
it keep saying username or password incorrect but is in database, then i can’t view the index and other pages apart from login page
Make sure your database connection is working, this tutorial have my credentials, so make sure you have updated these credentials in db.php with your credentials.
what do you mean credentials? Need any payment?
Credentials are your database user, password, host and database name.
i can’t view the index and other pages apart from login page, same problem as previous user
Make sure you are using php version 5.6 at least.
why edit code not working for me even though no error is seen.
You need to debug the error, first check if database connection working on that page by printing something from database. Then debug other things such as form visible or not? getting GET value from the URL or not.
hi brother, I found your codes very useful to me as i’m learning php as a beginner.
The way you had coded is very easy to understand.
thankyou very much.
and, what I want to know is, can we set download database function from php and MySQL?
What do you mean by download database function?
Mr Javed what is the uses of dashbord code ccould you tell me please!!!!!!
You can reach your logged in home when ever you click on dashboard and there you can place any important links to navigate.
I Would like to great thanks for you Mr Javed your tutorial is best
Dear Solomon, i am glad that you like it.
can you help me?? i created web site. but my php code is not working….
What kind of error you are getting and which page?
hey man im having some problems with edit page here
<input name="id" type="hidden" value="” />
<input type="text" name="name" placeholder="Enter Name"
required value="” />
it was supposed to show the current row name and nothing happens its just in blank
Make sure your database connection is working on this page, and simply fetch row or record to test if it is working or not.
hey man im having some problems with edit page in line 24
if(isset($_POST[‘new’]) && $_POST[‘new’]==1)
what’s the ‘new’ camp?
If you insert new record it checks if it is set new record is inserted.
Sir can u guide me that i want to update the data of particular row clicking on edit button and a modal box open on that click containing the current value and from there only i want to update the fields
I have created separate tutorials for popup and this tutorial is focus on CURD operations, you can simply check here how i updated record. And for popup you can check my other tutorial in demo section.
Can you share joins use php crud applications,sir
Satish, i didn’t use join here, you can get better examples of join in w3schools or other websites. Currently i didn;t write any tutorial about it.
can you add cancel button on registration.
What do you mean by cancel button? do you want to disable user?
If you want to disable user, simply add new column in user table with field name active, and put 1 for active user and 0 for disabled user.
Sir, very cute work you have here. please on the insert function is it possible to make users who uploaded the file to see only what they uploaded. and limit other users to insert and edit what another user uploaded?
how can i get that done????
Yes it is possible, everything is possible, you will need to insert data based on user ID and show only those results which are uploaded by same user ID, in this way, users will view only their uploaded data.
Hello Friend Javed
AOA
I want a complete form with master and detail as per below format. kindly send as soon as possible.
Thanks in advance.
Best regards,
Purchase No. : 0001 Date : 29/04/2019
Supplier Name : abc company
Ref No. : y-001
sr. no Prod. Code Product Name qty rate amount discount net amount
1 0001 Computer 10 20,000 200,000 5,000 195,000
2 0002 Printer 2 9,000 18,000 500 17,500
.more entries ======= ===== =======
. 218,000 5,500 212,500
What’s in auth.php?
You can see yourself that we are checking if user is logged in or not.
THANK YOU Mr JAVED I ALREADY REACHED MY DREAM BY FOLLOWING YOUR TUTORIALS.
I am glad that you found my tutorial helpful. 🙂
thank you it is very helful
You most welcome
Hi Javed, your code is excellent. It worked really smoothly, first time. For the Password Recovery code, there is a file index.php. There is also a similar one in the main program. Do I replace the first index.php file with the second one?
Also, in a separate project I am working on, I have a list of football matches, and next to each match row I want to add fields for the predicted scores in two separate fields(example: homescore 2, awayscore 2 and insert these to the database. I would like to have an Insert button and an Edit button, all in the same row. How do you suggest I go about it please?
I have separately shared forgot password tutorial, kindly check it. I hope that will help you out.
your blog such a helpful,thank you so much,
Thanks Mukesh, i am glad that you found it helpful. 🙂
Dear JAVED UR REHMAN,
Always you help people I benefit by your codes
wish you a brilliant future
Thanks Mohamed Saleh, I am glad that you find my scripts helpful. Thanks for the compliment.
I can retrieve records, I can go to insert, but it will not insert records, errors out. I cannot edit records, errors out. I can delete records no problem. Can I email you my code to look at? My database is for internal network, no external access.
Awesome tutorial never like anywhere online
Hello dear Javed Ur Rehman. Thank you for brilliant script. But how can changed that after inserting new record the script automatically show view.php instead view.php with “status” New record inserted? I do it with “header” sting but it’s not working. Thank you.
P.S. This problem i have with update record
<?php
if(isset($_POST['new']) && $_POST['new']==1)
{
$trn_date = date("Y-m-d H:i:s");
$name =$_REQUEST['name'];
$age = $_REQUEST['age'];
$submittedby = $_SESSION["username"];
$ins_query="insert into new_record (`trn_date`,`name`,`age`,`submittedby`) values ('$trn_date','$name','$age','$submittedby')";
mysqli_query($con,$ins_query) or die(mysql_error());
$status = "New Record Inserted Successfully. View Inserted Record”;
//– this line not work
header(“HTTP/1.1 301 Moved Permanently”);
header(“Location: view.php”);
}
?>
Kindly check my update query, you are using insert query.
Also header will redirect you only if there is no HTML generated before it. First test header redirect on separate page check is it working on plane page or not.
Thank you for fastest reply. It was my fault, sorry. I forgot to write “;” in my file… Your script work best. But your script have no pagination and this is a problem, because i have more than 200 records… And using your pagination script i have a problem. When i editing a record and click “submit button” the script reloading me to first page, but i’m editing for example a record on 15 page… Sorry for my bad english.
whats is this not receiving any email not you give full script on the site.A lot of issues with code
Can someone tell me why this is failing?
$update=”UPDATE Camera SET Site='”.$Site.”‘,Driver Nb='”.$DriverNb.”‘,Driver Name='”.$DriverName.”‘,FM='”.$FM.”‘,DLM='”.$DLM.”‘,SafetyRep='”.$SafetyRep.”‘,Issued='”.$Issued.”‘,Returned='”.$Returned.”‘,Camera Serial='”.$CameraSerial.”‘,Camera Status='”.$CameraStatus.”‘,Monitor Serial='”.$MonitorSerial.”‘,Monitor Status='”.$MonitorStatus.”‘,Comments='”.$Comments.”‘ WHERE id='”.$id.”‘”;
mysqli_query($conn,$update) or die(“failing at query: ” . mysqli_error());
Hi, thanks for the code, however, when I enter data into the insert page, it returns a blank page and the view records table does not reflect the entered data, how can I fix this, thank you
Hi Mike, make sure that your database connectivity is working fine, if your database is not connected then you will not be able to insert or fetch updated records.
Kudos to your great work, I got your source code on “insert, view, edit and delete Record from database using php and mysqli” after learning with it I tried uploading it in a live server but after registration and login, it will show a white screen instead of proceeding index.php page, please help me out, exams is at the corner
Try to move session start at the beginning of the page if you found it in the middle of code.
I am receiving the following error sometimes not all the time can you advise if i send you what i am doing please:
Dashboard | Insert New Record | Logout
Update Recipe Record
Warning: mysqli_error() expects exactly 1 parameter, 0 given in C:\xampp\htdocs\download1\demo\edit.php on line 64
you can see whats happening in the test website:
regards Steve Jones
I did check and i didn’t find anything wrong, i think you should check line no 64 and see what is missing over there, i think you should run my code separately first, if it is working separately fine then you should integrate it with your module.
Hi Javed Ur Rehman,
great tut. could you maybe do a simple username change page to work with this system?
For this purpose you must need to make email as unique ID and allow users to change their username, add additional column with name username that they can change easily based on user email address.
Unable to connect
Firefox can’t establish a connection to the server at localhost.
The site could be temporarily unavailable or too busy. Try again in a few moments.
If you are unable to load any pages, check your computer’s network connection.
If your computer or network is protected by a firewall or proxy, make sure that Firefox is permitted to access the Web.
I am getting this error.can u help me to solve it??
Richa browser does not have anything with the database connection, you need to enter your credentials in db.php file, if you do not know how to connect database using php, then you can search on Google for database connection examples.
Hi sir i’m a 3rd yr student! How to solve these type of errors from the above coding?
“”Warning: require(db.php): failed to open stream: No such file or directory in C:\xampp\htdocs\login\insert.php on line 2
Fatal error: require(): Failed opening required ‘db.php’ (include_path=’C:\xampp\php\PEAR’) in C:\xampp\htdocs\login\insert.php on line 2″”
its syntax error maybe .. use require(‘db.php’); .. hope it will work
Respected sir when i insert the name and age in insert.php it show the Warning: mysqli_error() expects exactly 1 parameter, 0 given in /opt/lampp/htdocs/php/insert.php on line 13.all code same as your.
please sir give the solution of this warning. Thanks
Make sure that your database is connected.
Javed I’m facing Error about the edit button.
<input name="ID" type="hidden" value="” />
<input type="text" name="Product" placeholder="Enter Product" required value="” />
<input type="text" name="Price" placeholder="Enter Age" required value="” />
<input type="text" name="Quantity" placeholder="Enter Age" required value="” />
When I run the html It suddenly go to Auth.php
It Seems like that you changed the names of input fields, first i will suggest to run the tutorial same as i shared with you, once you understanding working then you can modify it for your need.
HI Javed
help me
how to display data based on username
and how to maintain when the same username and email registration then can not register and there is a forgotten password menu
Well you can show data based on username, just keep relevant data in your database and compare username and show your data.
Forgot password can be reset, i have wrote tutorial about it here https://www.allphptricks.com/forgot-password-recovery-reset-using-php-and-mysql/
please give examples of queries from the tutorial above, because I just learned php
Well although i try my best to share simple tutorials, you can check more tutorials on my blog to understand more basic of PHP.
Hi Javed, love the tutorial.
In the ‘insert.php’ code, does the following code cause or have an effect on an undefined index below such as ‘name’:
if(isset($_POST[‘new’]) && $_POST[‘new’]==1){
Also does this need to be edited if I add more inputs to the form>?
Thanks in advance
No it does not need to be edit, you can any number of fields but if you remove the above condition so you may have to face undefined index issue, because it checks either form is submitted or not, if form is submitted then we get the values and insert them. If you remove it then when you will open this form it will try to find variables which are not available so you have this error.
That’s great thanks for the help!
Does this include protection against SQL injection?
Include Auth is checking is user logged in or not.
Thanks for clarifying I wasn’t sure if that counted as security against SQL injection.
Can you help me with a way of adding password hashing with password_hash and password_verify?
Javed Ur Rehman I have register user every time. But I am not able to login on your demo profile. Are you sure your code is working fine. Because I am a developer. Please check again and let me know what is the issue.
Thank you for share this code. But Please do correct…
Then it will very help full for another guy. I don’t mind
Thanks ..
Hi Rohan, Demo is working fine. Make sure that you are using the correct user and password which is mentioned below in the demo link.
I’m also facing the same problem
Bilal can you tell me what user and password you are entering in my online demo link?
Good Work!!!
Thanks a Lot!!!
You welcome 🙂
Warning: mysqli_fetch_assoc() expects parameter 1 to be mysqli_result, boolean given in /home/ubuntu/workspace/bearbeiten.php on line 17
I dont know how to fix this :/ can you help me plz ???
Probably you are facing database connection issue, kindly make sure that your database connection is working properly. To know more about database connection you can see example here https://www.w3schools.com/php/func_mysqli_fetch_assoc.asp
i got this error but i do not know how to solve this problem
Notice: Undefined index: ic in C:\xampp\htdocs\OnlineRegister\delete.php on line 4
There is no need to browser delete page, if you browse it will give error because we need id to get and use to delete data of that id, if you open this page directly it need id, and it is not able to find id therefore it will give you error, better you just use it in view page as i used.
Warning: mysqli_error() expects parameter 1 to be mysqli, string given in C:\xampp\htdocs\cnd\edit.php on line 35
Hi
I am getting this error on edit page
Make sure your database connection is working fine. Also mysqli is enabled on your host or localhost.
Thanks Buddy, It worked.
I am a java developer but trying to learn php as well. 🙂
This article is perfect for beginner.
You welcome Mrinal.
what does the $con stands for? what does it define ,it is found on the edit page and $result = mysqli_query($con,$sel_query);
while($row = mysqli_fetch_assoc($result)) { ?>
what does the above code fine?
i also have problems with the insert.php with this code ,it gives error saying something about column 1 and denies to insert data into database
$con is used for db connection, you can read more about it details on Google, db connection using mysqli.
How to display user data after login?
Such as name, email, address, contact no.
You can display anything from database, i am also showing information from database on view page, kindly check view page above for implementation example.
Dear Sir,
please send reply for bellow points.
how to i have md5 to string convert password in php and codignator.
and one site complete in WordPress but i have first open my static html page then redirect main site how to do it.
Using md5 function is very easy, you can check sample here https://www.w3schools.com/php/func_string_md5.asp
If you want show your main WordPress home as static then simply create Wp Page Template, put your all static content there and publish a new page with any name that you want, go to WP setting > Reading Settings and select that page as your homepage.
Instead of name and age, would like to have name and email,phone
Yes you can use any no. of fields, just add them in DB table and in your query. Like i did for these fields.
Thanks bro it really help God bless you.
your way of explaining is wonderful and practical. Every thing working well. My doubt is that using login code, it is not directing to any page. screen becomes clear and no link appears.
i am getting following error
[09-May-2018 18:43:08 Asia/Calcutta] PHP Warning: session_start(): Cannot send session cache limiter – headers already sent (output started at /home/pctant/public_html/editing_data/login.php:10) in /home/pctant/public_html/editing/login.php on line 12
Check your database connection, because if you got error in DB connection, it will print DB error message, which will appear before session_start(). SO if anything print before session_start() you will get these kind of error.
OK.Thanks a lot for your quick response. God Bless
Hi. I have Warning: session_start(): Cannot send session cache limiter – headers already sent (output started at /public_html/admin/login.php:1) in /public_html/admin/login.php on line 12
DB connection is fine
Well it seems like you are getting error in DB connection, therefore you are getting error of “headers already sent” which means db connection failed and it generated html code, therefore session_start() giving error.
Good day,
Please i am having this issue, i have a site with user account, when i log in as admin i cannot edit users profile.
I tried to add edit button on the profile page, but when i edit it does not change the value.
I added ModifyPhone.php, i edited process.php, also edited the profile index page, what else do i do so the change will be saved and reflect on the user details
Gary kindly check update query, as update query help you to update data in your table, better you should try your query on sql direct, if that is not updating in direct sql then you should first fix your sql query to reflect the update.
why i cannot view and update record
Well difficult to figure what is actually reason in your case, check your database connection.
can u help me do inventory system for ict equipment in hospital just do insert,view, update and delete function…
Dear Nurul, currently i do not having and such tutorial, however you can use my above code script for all insert, view, update and delete function, all you need is just to change fields name and data as per your need.
Thanks
ok thanks a lot 🙂
I just came to learn only Insert data on MySQL Database, but because of your guide, I learn easily to update database too.
Thank you Javed.
Big Thumbs Up👍👍👍👍👍
Thanks Riyaz, share it with others. 🙂
when i try to log in then is shows me this error plz help me
mysqli_error() expects exactly 1 parameter, 0 given in C:\xampp\htdocs\php crud\login.php on line 28
Thanks a lot for the time and effort you put in to make this tutorial easy to understand. I really benefited from it. Please I have one question. Everything is working fine but it still shows undefined parameter. Mysqli requires one parameter. It shows it on the edit page.. Could it be because I’m not yet logged in?
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ” where id=’1” at line 3
i got this arrow. can u explain it ?
Thanks a lot buddy,I really needed this,
All works well
Thanks Henry 🙂
Very useful article
I am glad that you found it helpful, share with others.
I like your code man. You are great.
Good Job.
But it seems you only fetch the last record in the databse to be display in the view.php using “ORDER BY id DESC.
Thanks.
1.Notice: Undefined index: id in C:\xampp\htdocs\practice\delete.php on line 3
Warning: mysqli_error() expects exactly 1 parameter, 0 given in C:\xampp\htdocs\practice\delete.php on line 5
HOW TO FIX IT But the code is same as ur
2.when i am running these modules it redirect me on the login page
how to fix it….
You can not directly open this page, as this page require an ID which record you want to delete, also you must be make sure that you are logged in to delete any record.
so how to fix the problem? Then, I should open from which page?
You will be able to see link in View Page, just click on that button from there.
is there any relation between the tables?
Thank you, this is very helpful.
This is great, thank you. How does this apply when working with radio buttons and dropdown menus?
thanks for this great tutorial but after login , it shows me a blank page ,
how to fix this issue
thanks
Check if your session is set or not, at the time of logged in $_SESSION[‘username’] is set.
Also make sure you are redirected to the index page, check the address bar URL must contain /index.php
if you do not see index.php after log in this mean you are not redirecting.
I will advise you to check this code on both local machine and web server, sometimes this happen due to PHP version issue.
Can you direct me to a page that will show me how to NOT duplicate email addresses when users try to sign up? In other words, if the email is already in database show error and reset their password if they like. Thank you for great tutorial.
Thankyou sir !
i want to let you know that i have tried several tutorials but this your tutorial is the BEST , I mean THE BEST , no error, no hidden code, , man You are good, May God bless you for me
Very interesting tutorial, I’m wondering for instance if user1 can edit or delete the post of user2.
When you are creating user…it creates
when another creates the same username it blocks both id???
solution please????
im stucked there there first command i dont know where to type that command
That is a table creation command…..
1. Create Another Table for Records
Execute the below SQL query:
CREATE TABLE IF NOT EXISTS `new_record` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`trn_date` datetime NOT NULL,
`name` varchar(50) NOT NULL,
`age`int(11) NOT NULL,
`submittedby` varchar(50) NOT NULL,
PRIMARY KEY (`id`)
);
1
2
3
4
5
6
7
8
CREATE TABLE IF NOT EXISTS `new_record` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`trn_date` datetime NOT NULL,
`name` varchar(50) NOT NULL,
`age`int(11) NOT NULL,
`submittedby` varchar(50) NOT NULL,
PRIMARY KEY (`id`)
);
Please how do i do this command in cmd or where??
In your php backend table creation point or try this to your php insert query page…..
sir i want a code in mysqli in which login/logout time will be save in databse.please help sir.
Are you sure the insert code is correct?? seems to have no relation to the database ‘registers’ that we create at start of tutorial .
Hi i cant get your connect db to even work so i changed with one i use and it connected.,then every page is full of errors like:(view page)
mysqli_fetch_assoc() expects parameter 1 to be mysqli_result, boolean given in C:\wamp64
and in (insert page)
Parse error: syntax error, unexpected ‘$trn_date’ (T_VARIABLE) in C:\wamp64 etc
looks really good as well,,,pity i cant get it to run smoothly.
thanks a lot
Thanks for your great article. It cleared my many doubts. As did all as you mentioned but after creating a user when i click submit and login it stays on login.php page and shows whole page white. it doesnt go to index.php page. Could you please help me this as i am stuck at this stage.
the same it happen to me ,please how to fix that
10Q it is helpfull but in my case insert.php is not working .
Pares error in line 12
Thanks for this tutorial i understand well and i can try to practice
goooood one bro,, you are a genius keep it up.. how i wish i could meet with you bro…
Very deep thanks Javed
you help me more
im mohamed saleh from sudan
thanks..
hi, first off, thank you for the clear code. One problem though, when I try to add a user and click the submit button, it goes to a blank page but does not add the user. I have tried to look for the problem for two days now with no success. kindly help.
As Salam Alaikum, do you think it is possible for there to be a page where the user may only be able to modify their own details? e.g. change only their own password.
simple create a member secure area and then users can edit anything you want to edit
It is great effort without any confusion. Pls continue your contribution
Thank u so much
Very Good and informative Article Tanks,
Please add pagination also. Thanks
This blog is very helpful for php beginner’s, i really appreciate with you tutorial….
Thanks
I am glad that you find it helpful 🙂
thank you very much for helping me out start over again with latest mysqli command. if any inquiry may i know your email?
I am very new to PHP and MySQL, just copied your code and run everything works perfectly fine. Please can you post code on how to insert more than two records into the Register database?
I made attempt to add more columns as Gender, Phone Number, Address, etc to be displayed when view.php was executed but ended up getting error message. Please assist.
Hello, I have noticed that it is possible to delete a record even though it is not logged in, because you do not call auth.php, so just enter the url and any ID and the record will be deleted by external people. It’s easy to fix but it’s important to warn. Sorry for spelling. Thanks, Alexandre Schmitz
Thank you so much, brother..Very great and awesome!
This article is great
Strict Standards: date() [function.date]: It is not safe to rely on the system’s timezone settings. Please use the date.timezone setting, the TZ environment variable or the date_default_timezone_set() function. In case you used any of those methods and you are still getting this warning, you most likely misspelled the timezone identifier. We selected ‘UTC’ for ‘8.0/no DST’ instead in C:\AppServ\www\file1\edit.php on line 27
how to solve this one/
Here is solution of your problem. http://stackoverflow.com/questions/5696833/it-is-not-safe-to-rely-on-the-systems-timezone-settings
It is great effort without any confusion. Pls continue your contribution
Thanks indeed
Thanks Kelum, i am glad that you find my tutorial helpful, share it with your friends 🙂
Your script is brilliant, straight forward keep it up.
can you add a search form?
I hope i will try to share that one also, but for now you can search at Google.
Great and clear, please help me how to create referral system to plug on my website?
Thanks that you like it but i didn’t write any tutorial about it, may be later, for now you can search it on Google.
super boss thank you so much!!